ajax技术现在越来越受到大家的欢迎,因为它能很好的解决一些以前不好解决的问题,动态检查文本框中的数据就是体现之一。现在已经加上了数据库mysql,可以说得上是一个比较完整的例子了。
下面就是怎样实现动态检查文本框中的数据一个例子:
工程目录如下:
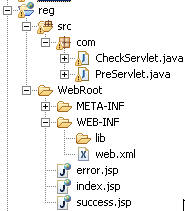
CheckServlet.java:
/**
*
*/
package com;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* @author Administrator
*
*/
public class CheckServlet extends HttpServlet {
public String[] usernameList;
public CheckServlet() {
super();
// TODO Auto-generated constructor stub
}
private boolean IsContain(String param){
for(int i=0;i<usernameList.length;i++){
if(usernameList[i].equals(param)){
return true;
}else{
continue;
}
}
return false;
}
public void doGet(HttpServletRequest request,HttpServletResponse response)throws IOException,ServletException{
String username=(String)request.getParameter("username");
String password=(String)request.getParameter("password");
String repassword=(String)request.getParameter("repassword");
String email=(String)request.getParameter("email");
if(username.equals("")||username==null){
request.setAttribute("error.message","用户名不能为空!");
RequestDispatcher requsetDispatcher=request.getRequestDispatcher("error.jsp");
requsetDispatcher.forward(request,response);
}else if(password.equals("")||password==null){
request.setAttribute("error.message","密码不能为空!");
RequestDispatcher requsetDispatcher=request.getRequestDispatcher("error.jsp");
requsetDispatcher.forward(request,response);
}else if(!password.equals(repassword)){
request.setAttribute("error.message","两次输入的密码不一致!");
RequestDispatcher requsetDispatcher=request.getRequestDispatcher("error.jsp");
requsetDispatcher.forward(request,response);
}else if(this.IsContain(username)){
request.setAttribute("error.message","您输入的用户名已经存在!");
RequestDispatcher requsetDispatcher=request.getRequestDispatcher("error.jsp");
requsetDispatcher.forward(request,response);
}else{
RequestDispatcher requsetDispatcher=request.getRequestDispatcher("success.jsp");
requsetDispatcher.forward(request,response);
}
}
public void doPost(HttpServletRequest request,HttpServletResponse response)throws IOException,ServletException{
doGet(request,response);
}
public void init(ServletConfig config) throws ServletException{
usernameList=new String[]{"Tom","Jerry","Brain"};
}
}
PreServlet.java:
package com;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Iterator;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class PreServlet extends HttpServlet {
public List usernameList;
/**
* Constructor of the object.
*/
public PreServlet() {
super();
}
/**
* Destruction of the servlet. <br>
*/
public void destroy() {
super.destroy(); // Just puts "destroy" string in log
// Put your code here
}
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setHeader("Cache-Control","no-cache");
String username=(String)request.getParameter("user_name");
System.out.println(username);
String xml="<?xml version=\"1.0\" encoding=\"UTF-8\"?>";
if(username.equals("")||username==null){
xml+="<message><info>Username is required!</info></message>";
}
else if(this.IsContain(username)){
xml+="<message><info>The username has existes,Please choose other username!</info></message>";
}
else{
xml+="<message><info>Username is approved!</info></message>";
}
response.getWriter().write(xml);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request,response);
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occure
*/
public void init() throws ServletException {
// Put your code here
DBOperator dBOperator=new DBOperator();
usernameList=dBOperator.getUsernameList();
}
private boolean IsContain(String param){
//这里修改了以前的代码,现在是读取数据库的数据。
Iterator it=usernameList.iterator();
while(it.hasNext()){
if(it.next().toString().equals(param)){
return true;
}else{
continue;
}
}
return false;
}
}
DBOperator.java:
package com;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
public class DBOperator {
public DBOperator(){}
public Connection Connect(){
Connection con = null;
try {
Class.forName("com.mysql.jdbc.Driver").newInstance();
con = java.sql.DriverManager.getConnection("jdbc:mysql://localhost/test?useUnicode=true&characterEncoding=GBK","root","");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return con;
}
public List getUsernameList(){
Connection con=Connect();
List usernameList=new ArrayList();
try {
Statement stmt=con.createStatement();
ResultSet rst=stmt.executeQuery("select * from m_stuinfo");
while(rst.next()){
usernameList.add(rst.getString("m_stuinfo_name"));
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return usernameList;
}
}
index.jsp:
<%@ page language="java" contentType="text/html; charset=utf-8"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>注册</title>
<SCRIPT type="text/javascript">
var req;
function UsrNameCheck()
{
var username=document.getElementById('username').value;
var url="pre?user_name="+username;
if(window.XMLHttpRequest)
{
req=new XMLHttpRequest();
}else if(window.ActiveXObject)
{
req=new ActiveXObject("Microsoft.XMLHTTP");
}
if(req)
{
req.open("GET",url,true);
req.onreadystatechange=callback;
req.send(null);
}
function callback()
{
if(req.readyState == 4)
{
if(req.status == 200)
{
parseMessage();
}else{
alert("Not able to retrieve description"+req.statusText);
}
}else{
document.getElementById('check_username').innerHTML="正在验证用户名
";
}
}
function parseMessage()
{
var xmlDoc=req.responseXML.documentElement;
alert(xmlDoc);
var node=xmlDoc.getElementsByTagName('info');
document.getElementById('check_username').innerHTML=node[0].firstChild.nodeValue;
}
function Form_Submit()
{
if(regForm.username.value=="")
{
alert("用户名不能为空");
return false;
}else if(regForm.password.value=="")
{
alert("密码不能为空");
return false;
}else if(regForm.password.value!=regForm.repassword.value)
{
alert("两次输入的密码不一致");
return false;
}
regForm.submit();
}
}
</SCRIPT>
</head>
<body>
<div align="center">
<form name="regForm" method="post" action="/reg/regservlet">
<table width="70%" border="1">
<tr align="center">
<td colspan="2">用户注册</td>
</tr>
<tr>
<td width="24%" align="center">用户名: </td>
<td width="76%" ><input name="username" type="text" id="username" onBlur="UsrNameCheck()">
<SPAN id="check_username"></SPAN>
</td>
</tr>
<tr>
<td align="center">密码: </td>
<td ><input name="password" type="password" id="password"></td>
</tr>
<tr>
<td align="center">重复密码: </td>
<td ><input name="repassword" type="password" id="repassword"></td>
</tr>
<tr>
<td align="center">email: </td>
<td ><input name="email" type="text" id="email"></td>
</tr>
<tr align="center">
<td colspan="2"><input type="button" name="Submit" value="提交" onClick="Form_Submit()"></td>
</tr>
</table>
</form>
</div>
</body>
</html> success.jsp:
<%@ page language="java" contentType="text/html; charset=GB2312"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=GB2312">
<title>注册成功</title>
</head>
<body>
恭喜你,注册成功!<br>
</body>
</html>
error.jsp:
<%@ page language="java" contentType="text/html; charset=GB2312"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=GB2312">
<title>失败</title>
</head>
<body>
<div align="center">
<table width="70%" border="1">
<tr>
<td>注册失败</td>
</tr>
<tr>
<td> <%=(String)request.getAttribute("error.message") %></td>
</tr>
<tr>
<td><a href="index.jsp">返回注册页</a></td>
</tr>
</table>
</div>
</body>
</html>
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>reg</display-name>
<!-- Action Servlet Configuration -->
<servlet>
<servlet-name>regservlet</servlet-name>
<servlet-class>com.CheckServlet</servlet-class>
</servlet>
<servlet>
<servlet-name>PreServlet</servlet-name>
<servlet-class>com.PreServlet</servlet-class>
</servlet>
<!-- Action Servlet Mapping -->
<servlet-mapping>
<servlet-name>regservlet</servlet-name>
<url-pattern>/regservlet</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>PreServlet</servlet-name>
<url-pattern>/pre</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
运行结果是:
posted on 2006-05-16 13:11
千山鸟飞绝 阅读(3686)
评论(6) 编辑 收藏 所属分类:
Ajax