这个一个ajax的经典示例,也是ajax的长处所在。不多说了,下面来看代码。
项目结构图:
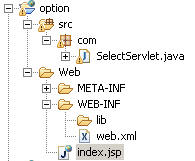
index.jsp:
<%@ page language="java" contentType="text/html; charset=utf-8"%>
<html>
<head>
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<SCRIPT type="text/javascript">
var req;
window.onload=function(){
}
function Change_Select()
{
var zhi=document.getElementById('hero').value;
var url="select?id="+escape(zhi);
if(window.XMLHttpRequest)
{
req=new XMLHttpRequest();
}else if(window.ActiveXObject)
{
req=new ActiveXObject("Microsoft.XMLHTTP");
}
if(req)
{
req.open("GET",url,true);
req.onreadystatechange=callback;
req.send(null);
}
}
function callback()
{
if(req.readyState == 4)
{
if(req.status == 200)
{
parseMessage();
}else{
alert("Not able to retrieve description"+req.statusText);
}
}
}
function parseMessage()
{
var xmlDoc=req.responseXML.documentElement;
var xSel=xmlDoc.getElementsByTagName('select');
var select_root=document.getElementById('skill');
select_root.options.length=0;
for(var i=0;i<xSel.length;i++)
{
var xValue=xSel[i].childNodes[0].firstChild.nodeValue;
var xText=xSel[i].childNodes[1].firstChild.nodeValue;
var option=new Option(xText,xValue);
try{
select_root.add(option);
}catch(e){
}
}
}
</SCRIPT>
</head>
<body>
<div align="center">
<form name="form1" method="post" action="">
<TABLE width="70%" boder="0" cellspacing="0">
<TR>
<TD align="center">Double Select Box</TD>
</TR>
<TR>
<TD>
<SELECT name="hero" id="hero" onChange="Change_Select()">
<OPTION value="0">Unbounded</OPTION>
<OPTION value="1">D.K.</OPTION>
<OPTION value="2">NEC.</OPTION>
<OPTION value="3">BOSS</OPTION>
</SELECT>
<SELECT name="skill" id="skill">
<OPTION value="0">Unbounded</OPTION>
</SELECT>
</TD>
</TR>
<TR><td> </td></TR>
</TABLE>
</form>
</div>
</body>
</html>
SelectServlet.java:
package com;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class SelectServlet extends HttpServlet {
/**
* Constructor of the object.
*/
public SelectServlet() {
super();
}
/**
* Destruction of the servlet. <br>
*/
public void destroy() {
super.destroy(); // Just puts "destroy" string in log
// Put your code here
}
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setHeader("Cache-Control","no-cache");
String targetId=request.getParameter("id").toString();
String xml_start="<selects>";
String xml_end="</selects>";
String xml="";
if(targetId.equalsIgnoreCase("0")){
xml="<select><value>0</value><text>Unbounded</text></select>";
}else if(targetId.equalsIgnoreCase("1")){
xml="<select><value>1</value><text>Mana Burn</text></select>";
xml +="<select><value>2</value><text>Death Coil</text></select>";
xml +="<select><value>3</value><text>Unholy Aura</text></select>";
xml +="<select><value>4</value><text>Unholy Fire</text></select>";
}else if(targetId.equalsIgnoreCase("2")){
xml="<select><value>1</value><text>Corprxplode</text></select>";
xml +="<select><value>2</value><text>Raise Dead</text></select>";
xml +="<select><value>3</value><text>Brilliance Aura</text></select>";
xml +="<select><value>4</value><text>Aim Aura</text></select>";
}else{
xml="<select><value>1</value><text>Rain of Chaos</text></select>";
xml +="<select><value>2</value><text>Finger of Death</text></select>";
xml +="<select><value>3</value><text>Bash</text></select>";
xml +="<select><value>4</value><text>Summon Doom</text></select>";
}
String last_xml=xml_start+xml+xml_end;
response.getWriter().write(last_xml);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request,response);
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occure
*/
public void init() throws ServletException {
// Put your code here
}
}
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<servlet>
<servlet-name>SelectServlet</servlet-name>
<servlet-class>com.SelectServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>SelectServlet</servlet-name>
<url-pattern>/select</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
运行结果图:
posted on 2006-05-17 11:10
千山鸟飞绝 阅读(24014)
评论(25) 编辑 收藏 所属分类:
Ajax