Client端:
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.Scanner;
public class ChatClient {
private static final String SERVER_IP = "127.0.0.1";
private static final int SERVER_PORT = 8888;
Socket socket = null;
DataOutputStream dos = null;
DataInputStream dis = null;
private boolean bConnected = false;
private static Scanner in = new Scanner(System.in);
private static String hostInfo = null;
Thread tRecv = new Thread(new RecvThread());
public static void main(String[] args) {
new ChatClient().launch();
}
public void launch() {
connect();
tRecv.start();
}
public void connect() {
try {
socket = new Socket(SERVER_IP, SERVER_PORT);
dos = new DataOutputStream(socket.getOutputStream());
dis = new DataInputStream(socket.getInputStream());
bConnected = true;
InetAddress addr = InetAddress.getLocalHost();
String ip = addr.getHostAddress().toString();// 获得本机IP
String address = addr.getHostName().toString();// 获得本机名称
hostInfo = ip + "\t" + address + "\tconnected.";
System.out.println(hostInfo);
dos.writeUTF(hostInfo);
dos.flush();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public void disconnect() throws IOException {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
}
}
private class RecvThread implements Runnable {
public void run() {
while (bConnected) {
try {
if (in.hasNext()) {
String talk = in.nextLine();
if (talk.equals("quit")) {
disconnect();
bConnected = false;
break;
}
dos.writeUTF(talk);
dos.flush();
}
String talk = dis.readUTF();
System.out.println(talk);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
server端:
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.EOFException;
import java.io.IOException;
import java.net.BindException;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
public class ChatServer {
boolean started = false;
ServerSocket ss = null;
List<Client> clients = new ArrayList<Client>();
public static void main(String[] args) {
new ChatServer().start();
}
public void start() {
try {
ss = new ServerSocket(8888);
started = true;
} catch (BindException e) {
System.err.println("port in use.please stop program using this port and restart.");
System.exit(0);
} catch (IOException e) {
e.printStackTrace();
}
try {
while(started) {
Socket s = ss.accept();
Client c = new Client(s);
// System.out.println("a client connected.");
new Thread(c).start();
clients.add(c);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Client implements Runnable {
private Socket s;
private DataInputStream dis = null;
private DataOutputStream dos = null;
private boolean bConnected = false;
public Client(Socket s) {
this.s = s;
try {
dis = new DataInputStream(s.getInputStream());
dos = new DataOutputStream(s.getOutputStream());
bConnected = true;
} catch (IOException e) {
e.printStackTrace();
}
}
public void send(String str) {
try {
dos.writeUTF(str);
dos.flush();
} catch (IOException e) {
clients.remove(this);
System.out.println("a client quit.");
//e.printStackTrace();
}
}
public void run() {
try {
while(bConnected) {
String str = dis.readUTF();
solveTalk(str);
}
} catch (EOFException e) {
System.out.println("Client closed!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(dis != null) dis.close();
if(dos != null) dos.close();
if(s != null) {
s.close();
s = null;
}
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
private void solveTalk(String talk) {
System.out.println(talk);
for(int i=0;i<clients.size();i++) {
Client c = clients.get(i);
c.send(talk);
}
}
}
}
posted @
2015-08-18 10:03 marchalex 阅读(371) |
评论 (0) |
编辑 收藏
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegexTestHarnessV4 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(
new InputStreamReader(new BufferedInputStream(System.in))
);
while (true) {
System.out.print("\nEnter your regex: ");
Pattern pattern = Pattern.compile(br.readLine());
System.out.print("Enter input string to search: ");
Matcher matcher = pattern.matcher(br.readLine());
boolean found = false;
while (matcher.find()) {
System.out.println("I found the text \"" + matcher.group() +
"\" starting at index " + matcher.start() +
" and ending at index " + matcher.end() +
".");
found = true;
}
if (!found) {
System.out.println("No match found.");
}
}
}
}
样例:
Enter your regex: [0-9]+
Enter input string to search: fdsdffd9090fd
I found the text "9090" starting at index 7 and ending at index 11.
Enter your regex: class="fav-num[^"]*"[^>]*>[^<]*
Enter input string to search: <i class="litb-icon-fav-on"></i><i class="litb-icon-fav-off"></i><span class="fav-num">(151)</span>
I found the text "class="fav-num">(151)" starting at index 71 and ending at index 92.
资料:
http://blog.csdn.net/yaerfeng/article/details/28855587
posted @
2015-08-12 13:58 marchalex 阅读(324) |
评论 (0) |
编辑 收藏
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class GoogleFinderNew {
private static String address = "https://www.google.com.hk/search?hl=en&q=";
private static String query = "";
private static String charset = "UTF-8";
private static List<String> useragentList = new ArrayList<String>();
private static void initUserAgentList(String filename) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line = null;
while((line = reader.readLine()) != null){
useragentList.add(line.trim());
}
reader.close();
return;
}
private static List<String> getpages(URL url) throws IOException {
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String line;
String ans = "";
while ((line = reader.readLine()) != null){
ans += line + "\n";
}
int st = -1, ed = 0;
List<String> pagesList = new ArrayList<String>();
while((st = ans.indexOf("<h3 class=\"r\"><a href=\"", ed)) != -1) {
ed = ans.indexOf("\"", st+23);
//System.out.println(ans.substring(st+23, ed));
pagesList.add(ans.substring(st+23, ed));
}
return pagesList;
}
public static void main(String[] args) throws MalformedURLException, IOException, InterruptedException {
Scanner in = new Scanner(System.in);
String askurl = in.nextLine();
query = in.nextLine();
initUserAgentList("D:\\useragent.txt");
//System.setProperty("http.agent", "Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US) AppleWebKit/525.19 (KHTML, like Gecko) Chrome/0.3.154.9 Safari/525.19");
int pages = 10;
for(int i=0;i<pages;i++) {
System.out.println((i+1) + " ..");
int index = (int)(useragentList.size()*Math.random());
if(index == useragentList.size()) index --;
String theUserAgent = useragentList.get(index);
System.setProperty("http.agent", theUserAgent);
String urlString = address + URLEncoder.encode(query, charset);
if(i != 0) urlString += "&start=" + i + "0";
//System.out.println(urlString);
List<String> list = getpages(new URL(urlString));
for(String page : list) {
if(page.contains(askurl)) {
// if(page.equals(askurl)) {
System.out.println(askurl + " found in the " + (i+1) + " th page.");
System.out.println(page);
return;
}
}
int extraTime = 0; //(int)(3000 * Math.random());
Thread.sleep(1000 + extraTime);
}
System.out.println("can't find " + askurl + " in the first " + pages + " pages.");
}
}
posted @
2015-07-29 16:41 marchalex 阅读(277) |
评论 (0) |
编辑 收藏
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.List;
import java.util.Scanner;
import java.util.StringTokenizer;
import com.google.gson.Gson;
public class GoogleFinder {
public static void main(String[] args) throws IOException, InterruptedException {
String address = "http://ajax.googleapis.com/ajax/services/search/web?v=1.0&q=";
String query = "";
String charset = "UTF-8";
Scanner in = new Scanner(System.in);
String targeturl = in.nextLine();
query = in.nextLine();
for(int i=0;i<125;i++) {
URL url = new URL(address + URLEncoder.encode(query, charset) + "&start=" + i);
//System.out.println(url);
Reader reader = new InputStreamReader(url.openStream(), charset);
GoogleResults results = new Gson().fromJson(reader, GoogleResults.class);
for(int t=0;t<30;t++) {
System.out.print(".");
Thread.sleep(2000);
} System.out.println("");
for(int j=0;j<4;j++) {
String ss = results.getResponseData().getResults().get(j).getUrl().toLowerCase();
if(ss.contains(targeturl)) {
System.out.println(ss);
System.out.println(results.getResponseData().getResults().get(j).getTitle());
System.out.println("Found in the " + (i*4+j+1) + " th");
return;
}
}
System.out.println("" + ((i+1)*4) + " pages found.");
}
System.out.println("Can find " + targeturl + " in the first 500 pages");
}
}
class GoogleResults {
private ResponseData responseData;
public ResponseData getResponseData() {
return responseData;
}
public void setResponseData(ResponseData responseData) {
this.responseData = responseData;
}
public String toString() {
return "ResponseData[" + responseData + "]";
}
static class ResponseData {
private List<Result> results;
public List<Result> getResults() {
return results;
}
public void setResults(List<Result> results) {
this.results = results;
}
public String toString() {
return "Results[" + results + "]";
}
}
static class Result {
private String url;
private String title;
public String getUrl() {
return url;
}
public String getTitle() {
return title;
}
public void setUrl(String url) {
this.url = url;
}
public void setTitle(String title) {
this.title = title;
}
public String toString() {
return "Result[url:" + url + ",title:" + title + "]";
}
}
}
posted @
2015-07-29 14:16 marchalex 阅读(293) |
评论 (0) |
编辑 收藏
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class GoogleFinderNew {
private static String address = "https://www.google.com.hk/search?hl=en&q=";
private static String query = "";
private static String charset = "UTF-8";
private static void test() {
String ans = "abc<h3>helloworld</h3>hehe<h3>nicetomeetyou</h3>";
int st = ans.indexOf("<h3>");
int ed = ans.indexOf("</h3>");
System.out.println(st + " " + ed);
System.out.println(ans.substring(st+4, ed));
}
private static List<String> getpages(URL url) throws IOException {
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String line;
String ans = "";
while ((line = reader.readLine()) != null){
ans += line + "\n";
}
int st = -1, ed = 0;
List<String> pagesList = new ArrayList<String>();
while((st = ans.indexOf("<h3 class=\"r\"><a href=\"", ed)) != -1) {
ed = ans.indexOf("\"", st+23);
//System.out.println(ans.substring(st+23, ed));
pagesList.add(ans.substring(st+23, ed));
}
return pagesList;
}
public static void main(String[] args) throws MalformedURLException, IOException, InterruptedException {
Scanner in = new Scanner(System.in);
String askurl = in.nextLine();
query = in.nextLine();
System.setProperty("http.agent", "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/536.11 (KHTML, like Gecko) Chrome/20.0.1132.11 TaoBrowser/2.0 Safari/536.11");
int pages = 100;
for(int i=0;i<pages;i++) {
System.out.println((i+1) + " ..");
String urlString = address + URLEncoder.encode(query, charset);
if(i != 0) urlString += "&start=" + i + "0";
//System.out.println(urlString);
List<String> list = getpages(new URL(urlString));
for(String page : list) {
if(page.contains(askurl)) {
// if(page.equals(askurl)) {
System.out.println(askurl + " found in the " + (i+1) + " th page.");
System.out.println(page);
return;
}
}
Thread.sleep(1000);
}
System.out.println("can't find " + askurl + " in the first " + pages + " pages.");
}
}
posted @
2015-07-29 14:16 marchalex 阅读(312) |
评论 (0) |
编辑 收藏
图片放在D:/pic/的p1.png,p2.png,p3.png,台词在D盘的lines.txt
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.StringTokenizer;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class GameFrame extends JFrame {
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static String content = null;
public GameFrame() throws Exception {
frame = new JFrame("");
frame.setLocationRelativeTo(null);
frame.setSize(Width, Height);
frame.setLocation(100, 30);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
BufferedReader reader = new BufferedReader(new FileReader("D:\\lines.txt"));
while((content=reader.readLine())!=null) {
JPanel panel = new GamePanel();
panel.setBounds(0, 0, Width, Height);
frame.getContentPane().add(panel);
frame.setVisible(true);
Thread.sleep(1000);
}
}
class GamePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
ImageIcon icon = new ImageIcon("D:\\pic\\bg.jpg");
g.drawImage(icon.getImage(), 0, 0, Width, Height, this);
g.setFont(new Font("", Font.PLAIN, 50));
StringTokenizer st = new StringTokenizer(content);
String name = st.nextToken();
if(name.equals("end")) {
g.setColor(Color.WHITE);
g.drawString("end", 470, 300);;
} else {
if(name.equals("p1")) {
icon = new ImageIcon("D:\\pic\\p1.png");
g.drawImage(icon.getImage(), 100, 50, 200, 300, this);
String words = st.nextToken();
g.setColor(Color.CYAN);
g.drawString(words, 100, 450);
} else if(name.equals("p2")) {
icon = new ImageIcon("D:\\pic\\p2.png");
g.drawImage(icon.getImage(), 700, 50, 200, 300, this);
String words = st.nextToken();
g.setColor(Color.PINK);
g.drawString(words, 500, 450);
} else if(name.equals("p3")) {
icon = new ImageIcon("D:\\pic\\p3.png");
g.drawImage(icon.getImage(), 450, 50, 200, 300, this);
String words = st.nextToken();
g.setColor(Color.GREEN);
g.drawString(words, 450, 450);
}
}
}
}
public static void main(String[] args) throws Exception {
new GameFrame();
}
}
posted @
2015-06-08 15:59 marchalex 阅读(307) |
评论 (0) |
编辑 收藏
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class FrameWork extends JFrame {
private static final double pi = Math.acos(-1.0);
private static final int Width = 1100;
private static final int Height = 650;
private static JFrame frame = null;
private static int the_time = -1095;
public FrameWork() {
frame = new JFrame("Frame");
frame.setLocationRelativeTo(null);
frame.setSize(Width, Height);
frame.setLocation(100, 30);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
for(int i=0;i<1000;i++) {
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, Width, Height);
frame.getContentPane().add(panel);
frame.setVisible(true);
the_time +=5;
}
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.WHITE);
g.fillRect(0, 0, Width, Height);
if(the_time < 0) {
g.setColor(Color.BLACK);
g.setFont(new Font("MS UI Gothic", Font.PLAIN, 50));
g.drawString(""+(-the_time/100), 50, 50);
}
int[] x = {500, 550, 150, 300, 800, 850, 950, 100};
int[] y = {500, 250, 200, 100, 190, 300, 450, 420};
g.setColor(Color.GRAY);
for(int i=0;i<8;i++)
for(int j=i+1;j<8;j++) {
g.drawLine(x[i], y[i], x[j], y[j]);
}
int[][] d = new int[8][8];
for(int i=0;i<8;i++)
for(int j=0;j<8;j++)
d[i][j] = (int)Math.sqrt((x[i]-x[j])*(x[i]-x[j])+(y[i]-y[j])*(y[i]-y[j]));
int[] start = new int[8];
start[0] = 0;
for(int i=1;i<=4;i++) start[i] = start[i-1] + d[i-1][i];
int d_long = 0;
for(int i=1;i<8;i++) if(d[0][i] > d_long) d_long = d[0][i];
int end_time = start[4] + d[0][4] + d_long;
if(the_time <= 0) g.setColor(Color.YELLOW);
else if(the_time > end_time-d_long) g.setColor(Color.RED);
else g.setColor(Color.ORANGE);
g.fillRect(x[0]-15, y[0]-30, 30, 30);
g.setColor(Color.BLACK);
g.drawRect(x[0]-15, y[0]-30, 30, 30);
if(the_time <= 0) g.setColor(Color.YELLOW);
else if(the_time > end_time-d_long) g.setColor(Color.RED);
else g.setColor(Color.ORANGE);
g.fillOval(x[0]-30, y[0]-20, 60, 40);
g.setColor(Color.BLACK);
g.drawOval(x[0]-30, y[0]-20, 60, 40);
for(int i=1;i<=4;i++) {
if(the_time <= start[i]) g.setColor(Color.BLUE);
else g.setColor(Color.darkGray);
g.fillRect(x[i]-15, y[i]-15, 30, 30);
g.setColor(Color.BLACK);
g.drawRect(x[i]-15, y[i]-15, 30, 30);
}
for(int i=5;i<8;i++) {
g.setColor(Color.GREEN);
g.fillOval(x[i]-15, y[i]-15, 30, 30);
g.setColor(Color.BLACK);
g.drawOval(x[i]-15, y[i]-15, 30, 30);
}
for(int i=0;i<5;i++) {
if(the_time > start[i]) {
int left_time = the_time - start[i];
for(int j=0;j<8;j++) {
if(i == j) continue;
if(d[i][j] > left_time) {
int xx = x[i] + (int)((double)(x[j]-x[i])*left_time/(double)d[i][j]);
int yy = y[i] + (int)((double)(y[j]-y[i])*left_time/(double)d[i][j]);
g.setColor(Color.CYAN);
g.fillOval(xx-5, yy-5, 10, 10);
}
}
}
}
if(the_time>end_time-d_long) {
for(int i=1;i<8;i++) {
int left_time = the_time - end_time + d_long;
if(left_time > d[0][i]) {
if(i>4) {
g.setColor(Color.RED);
g.fillOval(x[i]-15, y[i]-15, 30, 30);
g.setColor(Color.BLACK);
g.drawOval(x[i]-15, y[i]-15, 30, 30);
} else {
g.setColor(Color.RED);
g.fillRect(x[i]-15, y[i]-15, 30, 30);
g.setColor(Color.BLACK);
g.drawRect(x[i]-15, y[i]-15, 30, 30);
}
} else {
int xx = x[0] + (int)((double)(x[i]-x[0])*left_time/(double)d[0][i]);
int yy = y[0] + (int)((double)(y[i]-y[0])*left_time/(double)d[0][i]);
g.setColor(Color.RED);
g.fillOval(xx-5, yy-5, 10, 10);
}
}
}
g.setColor(Color.WHITE);
g.setFont(new Font("MS UI Gothic", Font.PLAIN, 30));
for(int i=1;i<=4;i++) {
g.drawString(""+i, x[i]-7, y[i]+7);
}
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-06-08 01:55 marchalex 阅读(251) |
评论 (0) |
编辑 收藏
运行此程序需要添加一个jar包
下载地址
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.StringTokenizer;
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
public class FileToExcel {
public static void main(String[] args) throws Exception {
writeToExcel("D:\\output.txt", "D:\\output.xls");
System.out.println("finished!");
}
public static void writeToExcel(String inFile, String outExcel) throws Exception {
//打开文件
WritableWorkbook book= Workbook.createWorkbook(new File(outExcel));
//生成名为“第一页”的工作表,参数0表示这是第一页
WritableSheet sheet=book.createSheet("第一页",0);
//在Label对象的构造子中指名单元格位置是第一列第一行(0,0)
//以及单元格内容为test
//Label label=new Label(0,0,"测试");
//将定义好的单元格添加到工作表中
//sheet.addCell(label);
//jxl.write.Number number = new jxl.write.Number(1,0,789.123);
//sheet.addCell(number);
//jxl.write.Label s=new jxl.write.Label(1, 2, "三十三");
//sheet.addCell(s);
//写入数据并关闭文件
Label label = null;
String s1 = readFile(inFile);
StringTokenizer st1 = new StringTokenizer(s1, "\n");
for(int i=0;st1.hasMoreElements();i++) {
String s2 = st1.nextToken();
StringTokenizer st2 = new StringTokenizer(s2, " \t");
for(int j=0;st2.hasMoreElements();j++) {
String s = st2.nextToken();
label=new Label(j,i, "" + s);
sheet.addCell(label);
}
}
book.write();
book.close(); //最好在finally中关闭,此处仅作为示例不太规范
}
private static String readFile(String filename) throws Exception {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String ans = "", line = null;
while ((line = reader.readLine()) != null) {
ans += line + "\r\n";
}
reader.close();
return ans;
}
}
posted @
2015-04-15 22:11 marchalex 阅读(421) |
评论 (0) |
编辑 收藏
运行此程序需要添加一个jar包
下载地址
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.StringTokenizer;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
public class ExcelToFile {
public static void main(String[] args) throws Exception {
solve("D:\\input.xls", "D:\\output.txt");
System.out.println("finished!");
}
private static String readFile(String filename) throws Exception {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String ans = "", line = null;
while ((line = reader.readLine()) != null) {
ans += line + "\r\n";
}
reader.close();
return ans;
}
private static void writeFile(String content, String filename)
throws Exception {
BufferedWriter writer = new BufferedWriter(new FileWriter(filename));
writer.write(content);
writer.flush();
writer.close();
}
private static void solve(String xls_name, String outfile) throws Exception {
String ans = "";
File file = new File(xls_name);
String[][] result = getData(file, 2);
int rowLength = result.length;
for(int i=0;i<rowLength;i++) {
for(int j=0;j<result[i].length;j++) {
ans += result[i][j]+" ";
}
ans += "\r\n";
}
writeFile(ans, outfile);
}
/**
* 读取Excel的内容,第一维数组存储的是一行中格列的值,二维数组存储的是多少个行
* @param file 读取数据的源Excel
* @param ignoreRows 读取数据忽略的行数,比喻行头不需要读入 忽略的行数为1
* @return 读出的Excel中数据的内容
* @throws FileNotFoundException
* @throws IOException
*/
public static String[][] getData(File file, int ignoreRows)
throws FileNotFoundException, IOException {
List<String[]> result = new ArrayList<String[]>();
int rowSize = 0;
BufferedInputStream in = new BufferedInputStream(new FileInputStream(
file));
// 打开HSSFWorkbook
POIFSFileSystem fs = new POIFSFileSystem(in);
HSSFWorkbook wb = new HSSFWorkbook(fs);
HSSFCell cell = null;
for (int sheetIndex = 0; sheetIndex < wb.getNumberOfSheets(); sheetIndex++) {
HSSFSheet st = wb.getSheetAt(sheetIndex);
// 第一行为标题,不取
for (int rowIndex = ignoreRows; rowIndex <= st.getLastRowNum(); rowIndex++) {
HSSFRow row = st.getRow(rowIndex);
if (row == null) {
continue;
}
int tempRowSize = row.getLastCellNum() + 1;
if (tempRowSize > rowSize) {
rowSize = tempRowSize;
}
String[] values = new String[rowSize];
Arrays.fill(values, "");
boolean hasValue = false;
for (short columnIndex = 0; columnIndex <= row.getLastCellNum(); columnIndex++) {
String value = "";
cell = row.getCell(columnIndex);
if (cell != null) {
// 注意:一定要设成这个,否则可能会出现乱码
cell.setEncoding(HSSFCell.ENCODING_UTF_16);
switch (cell.getCellType()) {
case HSSFCell.CELL_TYPE_STRING:
value = cell.getStringCellValue();
break;
case HSSFCell.CELL_TYPE_NUMERIC:
value = String.format("%.2f", cell.getNumericCellValue());
if (HSSFDateUtil.isCellDateFormatted(cell)) {
Date date = cell.getDateCellValue();
if (date != null) {
value = new SimpleDateFormat("yyyy-MM-dd")
.format(date);
} else {
value = "";
}
} else {
value = new DecimalFormat("0").format(cell
.getNumericCellValue());
}
break;
case HSSFCell.CELL_TYPE_FORMULA:
// 导入时如果为公式生成的数据则无值
if (!cell.getStringCellValue().equals("")) {
value = cell.getStringCellValue();
} else {
value = cell.getNumericCellValue() + "";
}
break;
case HSSFCell.CELL_TYPE_BLANK:
break;
case HSSFCell.CELL_TYPE_ERROR:
value = "";
break;
case HSSFCell.CELL_TYPE_BOOLEAN:
value = (cell.getBooleanCellValue() == true ? "Y"
: "N");
break;
default:
value = "";
}
}
if (columnIndex == 0 && value.trim().equals("")) {
break;
}
values[columnIndex] = value.trim();
hasValue = true;
}
if (hasValue) {
result.add(values);
}
}
}
in.close();
String[][] returnArray = new String[result.size()][rowSize];
for (int i = 0; i < returnArray.length; i++) {
returnArray[i] = (String[]) result.get(i);
}
return returnArray;
}
}
posted @
2015-04-15 21:57 marchalex 阅读(222) |
评论 (0) |
编辑 收藏
RandomRectangles类中的X、Y和n分别代表生成举行的横坐标范围、纵坐标范围和个数。
FrameWork类用于演示。
RandomRectangle.java
public class RandomRectangles {
private static final int maxn = 1010;
private static double[] x = new double[maxn];
private static double[] y = new double[maxn];
private static double[] w = new double[maxn];
private static double[] h =new double[maxn];
private static double[] xmax = new double[maxn];
private static double[] ymax = new double[maxn];
private static double[] xx = new double[maxn];
private static double[] yy = new double[maxn];
private static double X = 1000;
private static double Y = 600;
private static int n = 2;
private static double[][] ans = new double[n][4];
private static int cmp(int i, int j) {
if(y[i] < y[j] || y[i] == y[j] && x[i] < x[j]) return -1;
return 1;
}
private static void sort(int l, int r) {
if(l == r) return;
int mid = (l + r) >> 1;
sort(l, mid);
sort(mid+1, r);
int i = l, j = mid + 1, k = l;
while(i <= mid || j <= r) {
if(i > mid) {
xx[k] = x[j];
yy[k++] = y[j++];
}
else if(j > r) {
xx[k] = x[i];
yy[k++] = y[i++];
}
else if(cmp(i,j) == -1) {
xx[k] = x[i];
yy[k++] = y[i++];
}
else {
xx[k] = x[j];
yy[k++] = y[j++];
}
}
for(i=l;i<=r;i++) {
x[i] = xx[i];
y[i] = yy[i];
}
return;
}
private static boolean create_points() {
for(int i=0;i<n;i++) {
xmax[i] = X;
ymax[i] = Y;
x[i] = Math.random() * X;
y[i] = Math.random() * Y;
}
sort(0, n-1);
for(int i=0;i<n-1;i++) {
if(y[i] == y[i+1] && x[i] == x[i+1]) return false;
}
return true;
}
public static double[][] getData() {
while(true) {
if(create_points() == true) break;
}
for(int i=0;i<n-1;i++) {
if(y[i] == y[i+1]) xmax[i] = x[i];
}
for(int i=0;i<n;i++) {
w[i] = Math.random() * (xmax[i] - x[i]);
for(int j=i+1;j<n;j++) {
if(x[j] >= x[i] && x[j] <= x[i]+w[i]) {
ymax[i] = y[j];
break;
}
}
h[i] = Math.random() * (ymax[i] - y[i]);
for(int j=i+1;j<n;j++) {
if(x[j] < x[i] && y[j] >= y[i] && y[j] <= y[i]+h[i]) {
xmax[j] = xmax[j] < x[j] ? xmax[j] : x[j];
}
}
}
for(int i=0;i<n;i++) {
ans[i][0] = x[i];
ans[i][1] = y[i];
ans[i][2] = w[i];
ans[i][3] = h[i];
}
return ans;
}
public static void main(String[] args) {
ans = getData();
System.out.println(ans[2][2]);
}
}
FrameWork.java
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
public class FrameWork extends JFrame {
private static final double pi = Math.acos(-1.0);
private static final int Width = 1200;
private static final int Height = 800;
private static JFrame frame = null;
public FrameWork() {
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setSize(Width, Height);
setResizable(false);
getContentPane().setLayout(null);
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, Width, Height);
getContentPane().add(panel);
setVisible(true);
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.white);
g.fillRect(0, 0, Width, Height);
g.setColor(Color.black);
double[][] ans = RandomRectangles.getData();
for(int i=0;i<ans.length;i++) {
double x0 = ans[i][0];
double y0 = ans[i][1];
double x1 = x0 + ans[i][2];
double y1 = y0 + ans[i][3];
System.out.println(x0 + " " + y0 + " " + x1 + " " + y1);
}
for(int i=0;i<ans.length;i++)
g.drawRect((int)ans[i][0], (int)ans[i][1], (int)ans[i][2], (int)ans[i][3]);
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-04-15 20:27 marchalex 阅读(382) |
评论 (0) |
编辑 收藏
线段树是一种二叉树结构,能够在O(logn)时间复杂度之内实现对数组中某一区间的增删改查的操作。
关于线段树的
详细解释。
今天我们涉及的是线段树的单点更新以及区间查询功能。
我们以HDU上面的
敌兵布阵为例。
题目描述:
C国的死对头A国这段时间正在进行军事演习,所以C国间谍头子Derek和他手下Tidy又开始忙乎了。A国在海岸线沿直线布置了N个工兵营地,Derek和Tidy的任务就是要监视这些工兵营地的活动情况。由于采取了某种先进的监测手段,所以每个工兵营地的人数C国都掌握的一清二楚,每个工兵营地的人数都有可能发生变动,可能增加或减少若干人手,但这些都逃不过C国的监视。
中央情报局要研究敌人究竟演习什么战术,所以Tidy要随时向Derek汇报某一段连续的工兵营地一共有多少人,例如Derek问:“Tidy,马上汇报第3个营地到第10个营地共有多少人!”Tidy就要马上开始计算这一段的总人数并汇报。但敌兵营地的人数经常变动,而Derek每次询问的段都不一样,所以Tidy不得不每次都一个一个营地的去数,很快就精疲力尽了,Derek对Tidy的计算速度越来越不满:"你个死肥仔,算得这么慢,我炒你鱿鱼!”Tidy想:“你自己来算算看,这可真是一项累人的工作!我恨不得你炒我鱿鱼呢!”无奈之下,Tidy只好打电话向计算机专家Windbreaker求救,Windbreaker说:“死肥仔,叫你平时做多点acm题和看多点算法书,现在尝到苦果了吧!”Tidy说:"我知错了。。。"但Windbreaker已经挂掉电话了。Tidy很苦恼,这么算他真的会崩溃的,聪明的读者,你能写个程序帮他完成这项工作吗?不过如果你的程序效率不够高的话,Tidy还是会受到Derek的责骂的.
Java代码:
import java.io.*;
public class Main {
private static final int maxn = 50050;
private static long[] sum = new long[maxn<<2];
private static long[] a = new long[maxn];
private static void pushup(int rt) {
sum[rt] = sum[rt<<1] + sum[rt<<1|1];
}
private static void build(int l, int r, int rt) {
if(l == r) {
sum[rt] = a[l];
return;
}
int mid = (l + r) >> 1;
build(l, mid, rt<<1);
build(mid+1, r, rt<<1|1);
pushup(rt);
}
private static void add(int pos, long value, int l, int r, int rt) {
if(l == r) {
sum[rt] += value;
return;
}
int mid = (l+r) >> 1;
if(pos <= mid) add(pos, value, l, mid , rt<<1);
else add(pos, value, mid+1, r, rt<<1|1);
pushup(rt);
}
private static long query(int L, int R, int l, int r, int rt) {
if(L <= l && r <= R) return sum[rt];
int mid = (l + r) >> 1;
long ans = 0;
if(L <= mid) ans += query(L, R, l, mid, rt<<1);
if(R > mid) ans += query(L, R, mid+1, r, rt<<1|1);
return ans;
}
public static void main(String[] args) throws IOException {
int T, n, cas = 1;
StreamTokenizer in = new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
in.nextToken();
T = (int)in.nval;
while(T > 0) {
T --;
out.println("Case " + cas + ":");
cas ++;
in.nextToken();
n = (int)in.nval;
for(int i=1;i<=n;i++) {
in.nextToken();
a[i] = (long)in.nval;
}
build(1, n, 1);
while(true) {
in.nextToken();
String order = (String)in.sval;
if(order.equals("End")) break;
else if(order.equals("Query")) {
in.nextToken();
int L = (int)in.nval;
in.nextToken();
int R = (int)in.nval;
long ans = query(L, R, 1, n, 1);
out.println(ans);
} else if(order.equals("Add")) {
in.nextToken();
int pos = (int)in.nval;
in.nextToken();
long val = (long)in.nval;
add(pos, val, 1, n, 1);
} else if(order.equals("Sub")) {
in.nextToken();
int pos = (int)in.nval;
in.nextToken();
long val = -(long)in.nval;
add(pos, val, 1, n, 1);
}
}
}
out.flush();
}
}
posted @
2015-03-26 08:19 marchalex 阅读(481) |
评论 (0) |
编辑 收藏
<!-- JSP中的指令标识 -->
<%@ page language="java" contentType="text/html;charset=gb2312" %>
<%@ page import="java.util.Date" %>
<!-- HTML标记语言 -->
<html>
<head><title>JSP页面的基本构成</title></head>
<body>
<center>
<!-- 嵌入的Java代码 -->
<% String today = new Date().toLocaleString(); %>
<!-- JSP表达式 -->
今天是:<%=today %>
<!-- HTML标记语言 -->
</center>
</body>
</html>
JSP中的指令标识:利用JSP指令可以使服务器按照指令的设置来执行和设置在整个JSP页面范围内有效的属性。
HTML标记语言:HTML标记在JSP页面中作为静态的内容,浏览器将会识别这些HTML标记并执行。
嵌入的Java代码片段:通过向JSP页面中嵌入Java代码,可以使页面生成动态的内容。
JSP表达式:JSP表达式主要用于数据的输出。它可以向页面输出内容以显示给用户,还可以用来动态地指定HTML标记中属性的值。
posted @
2015-03-24 14:03 marchalex 阅读(189) |
评论 (0) |
编辑 收藏
功能:通过按钮打开一个新窗口,并在新窗口的状态栏中显示当前年份。
(1)在主窗口中应用以下代码添加一个用于打开一个新窗口的按钮:
<input name="button" value="打开新窗口" type="button" onclick="window.open('newWindow.jsp', '', 'width=400, height=200, status=yes')">
(2)创建一个新的JSP文件,名称为newWindow.jsp,在该文件中添加以下用于显示当前年份的代码:
<script language="javascript">
var mydate = new Date();
var year = "现在是:" + mydate.getFullYear() + "年!";
document.write(year);
</script>
posted @
2015-03-24 13:35 marchalex 阅读(277) |
评论 (0) |
编辑 收藏
1.事件概述
JavaScript与Web页面之间的交互时通过用户操作浏览器页面时触发相关事件来实现的。例如:
在页面载入完毕时,将处罚load(载入)事件;
当用户单击按钮时,将触发按钮的click事件等。
用户响应某个事件而执行的处理程序称为事件处理程序。例如:
当用户单击按钮时,将触发按钮的事件处理程序onClick。
事件处理程序的两种分配方式
(1)在JavaScript中分配事件处理程序
例:
<img src="http://gi3.md.alicdn.com/bao/uploaded/i3/TB1tlxaHpXXXXXtaXXXXXXXXXXX_!!0-item_pic.jpg_430x430q90.jpg" id="aimage">
<script language="javascript">
var img = document.getElementById("aimage");
img.onclick = function() {
alert('单击了图片');
}
</script>
在页面中加入上面的代码并运行,则当单击图片aimage时,将弹出“单击了图片”对话框。
在JavaScript中分配时间处理程序时,事件处理程序名称必须小写,才能正确响应事件。
(2)在HTML中分配事件处理程序
例:
<img src="http://gi3.md.alicdn.com/bao/uploaded/i3/TB1tlxaHpXXXXXtaXXXXXXXXXXX_!!0-item_pic.jpg_430x430q90.jpg" onclick="alert('单击了图片');">
2.事件类型
UI事件:如load、unload、error、resize、scroll、select、DOMActive,是用户与页面上的元素交互时触发的。
焦点事件:如blur、DOMFocusIn、DOMFocusOut、focus、focusin、focusout,在元素获得或失去焦点的时候触发,这些事件当中,最为重要的是blur和focus,有一点需要引起注意,这一类事件不会发生冒泡!
鼠标与滚轮事件:如click、dblclick、mousedown、mouseenter、mouseleave、mousemove、mouseout、mouseover、mouseup,是当用户通过鼠标在页面执行操作时所触发的。
滚轮事件:mousewheel(IE6+均支持)、DOMMouseScroll(FF支持的,与mousewheel效果一样)。是使用鼠标滚轮时触发的。
文本事件:textInput,在文档中输入文本触发。
键盘事件:keydown、keyup、keypress,当用户通过键盘在页面中执行操作时触发。
合成事件:DOM3级新增,用于处理IME的输入序列。所谓IME,指的是输入法编辑器,可以让用户输入在物理键盘上找不到的字符。compositionstart、compositionupdate、compositionend三种事件。
变动事件:DOMsubtreeModified、DOMNodeInserted、DOMNodeRemoved、DOMAttrModified、DOMCharacterDataModified等,当底层DOM结构发生变化时触发。IE8-不支持。
变动名称事件:指的是当元素或者属性名变动时触发,当前已经弃用!
对于事件的基本类型,随着HTML5的出现和发展,又新增了HTML5事件、设备事件、触摸事件、手势事件等各种事件。
posted @
2015-03-24 10:58 marchalex 阅读(207) |
评论 (0) |
编辑 收藏
在JSP中引入JavaScript
1.在页面中直接嵌入JavaScript
<script language="javascript">...</script>
2.连接外部JavaScript
<script language="javascript" src="sample.js"></script>
变量的声明用var
var variable;
var variable = value;
写的函数
document.write(str);
函数的定义
function functionName([parameter1, parameter2, ...]) {
statements
[return expression]
}
元素的获取
document.getElementById(id);
posted @
2015-03-24 09:50 marchalex 阅读(146) |
评论 (0) |
编辑 收藏
Vector类常用方法:
add(int index, Object element);
addElementAt(Object obj, int index);
size();
elementAt(int index);
setElementAt(Object obj, int index);
removeElementAt(int index);
Vector类实例:实现创建空的Vector对象,并向其添加元素,然后输出所有元素。
<%@ page import="java.util.*" %>
<%
Vector v = new Vector(); //创建空的Vector对象
for(int i=0;i<3;i++) {
v.add(new String("福娃" + i));
}
v.remove(1); //移除索引位置为1的元素
//显示全部元素
for(int i=0;i<v.size();i++) {
out.println(v.indexOf(v.elementAt(i))+": "+v.elementAt(i));
}
%>
显示结果为:
0: 福娃0 1: 福娃2
posted @
2015-03-23 11:03 marchalex 阅读(194) |
评论 (0) |
编辑 收藏
Java容器之ArrayList类
List集合的实例化:
List<String> l = new ArrayList<String>(); //使用ArrayList类实例化List集合
List<String> l2 = new LinkedList<String>(); //使用LinkedList类实例化List集合
ArrayList常用方法:
add(int index, Object obj);
addAll(int, Collection coll);
remove(int index);
set(int index, Object obj);
get(int index);
indexOf(Object obj);
lastIndexOf(Object obj);
listIterator();
ListIterator(int index);
ArrayList示例:实现创建空的ArrayList对象,并向其添加元素,然后输出所有元素。
<%@ page import="java.util.*" %>
<%
List<String> list = new ArrayList<String>();
for(int i=0;i<3;i++) {
list.add(new String("福娃" + i));
}
list.add(1, "后添加的福娃");
//输出所有元素
Iterator<String> it = list.iterator();
while(it.hasNext()) {
out.println(it.next());
}
%>
输出结果为:
福娃0 后添加的福娃 福娃1 福娃2
LinkedList类的用法与ArrayList类类似。
posted @
2015-03-23 10:57 marchalex 阅读(174) |
评论 (0) |
编辑 收藏
JSP显示时间的小应用,用于显示时间并对不同时间显示不同提示。
如:我在写这篇博客时打开的效果如下:
温馨提示! |
现在时间为:2015-03-23 10:17:28 |
午休时间!正午好时光! |
代码:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.util.Date,java.text.*" %>
<%
Date nowday = new Date(); //获取当前时间
int hour = nowday.getHours(); //获取日期中的小时
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //定义日期格式化对象
String time = format.format(nowday); //将指定日期格式化为“yyyy-MM-dd HH:mm:ss”形式
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>获取当前时间的JSP程序</title>
</head>
<body>
<center>
<table border="1" width="300">
<tr height="30"><td align="center">温馨提示!</td></tr>
<tr height="80"><td align="center">现在时间为:<%= time %></td></tr>
<tr height="70">
<td align="center">
<!-- 以下为嵌入到HTML中的Java代码,用来生成动态的内容 -->
<%
if(hour >= 24 && hour < 5)
out.print("现在是凌晨!时间还很早,再睡一会儿吧!");
else if(hour >= 5 && hour < 10)
out.print("早上好!新的一天即将开始,您准备好了吗?");
else if(hour >= 10 && hour < 13)
out.print("午休时间!正午好时光!");
else if(hour >= 13 && hour < 18)
out.print("下午继续努力工作吧!");
else if(hour >= 18 && hour < 24)
out.print("已经是深夜了,注意休息哦!");
%>
</td>
</tr>
</table>
</center>
</body>
</html>
posted @
2015-03-23 10:19 marchalex 阅读(229) |
评论 (0) |
编辑 收藏
Crawler类能够通过宽度优先搜索不断地抓取网站上的url。
这里需要用到
FileHelper类的writeFile方法用于写入文件。
代码如下:
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
public class Crawler {
private static HashMap<String, Integer> map =
new HashMap<String, Integer>();
private static int count = 0;
private static int max_count = 200000;
public static String[] getLinks(String content) {
HashMap<String, Integer> map =
new HashMap<String, Integer>();
int len = content.length();
for(
int i=0;i+9 < len;i++) {
if(content.substring(i, i+8).equals("\"http:
//") || content.substring(i, i+9).equals("\"https://")) {
String ss =
new String();
for(
int j=i+1;j<len && content.charAt(j) != '\"';j++) ss += content.charAt(j);
if(map.containsKey(ss))
continue;
map.put(ss,
new Integer(1));
}
}
int N = map.size();
String[] ans =
new String[N];
Iterator<String> iter = map.keySet().iterator();
int cnt = 0;
while (iter.hasNext()) {
String key = iter.next();
ans[cnt++] = key;
}
return ans;
}
private static boolean isPictureUrl(String url) {
int len = url.length();
if(url.substring(len-4, len).equals(".jpg")
|| url.substring(len-4, len).equals(".png")
|| url.substring(len-4, len).equals(".gif"))
return true;
return false;
}
public static void bfs(String u, String filename) {
String ans = "";
Queue<String> queue =
new LinkedList<String>();
map.put(u,
new Integer(1));
count ++;
queue.offer(u);
while ((u = queue.poll()) !=
null) {
System.out.println("digging in " + u);
System.out.println("have digged " + count + " pages now

");
String content;
try {
content = URLAnalysis.getContent(u);
String[] res = getLinks(content);
int n = res.length;
for (
int i = 0; i < n; i++) {
String v = res[i];
if (map.containsKey(v))
continue;
count ++;
ans += v + "\n";
map.put(v,
new Integer(1));
if(
false == isPictureUrl(v))
queue.offer(v);
}
if(count >= max_count)
break;
}
catch (Exception e) {
e.printStackTrace();
}
}
try {
FileHelper.writeFile(ans, filename);
}
catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
bfs("http://www.163.com", "D:\\test321\\urls.txt");
}
}
下面是部分输出内容:
http://
http://focus.news.163.com/15/0319/10/AL2INPO400011SM9.html
http://lady.163.com/15/0317/14/AKTR681900264IJ2.html
http://dajia.163.com/article/147.html#AL1GT1GU0095004J
http://xf.house.163.com/qhd/search/0-0-0-0-0-0-0-0-1.html
http://rd.da.netease.com/redirect?t=mwGQ3t&p=EA7B9E&target=http%3A%2F%2Fwww.kaola.com
http://tech.163.com/15/0321/07/AL7C7U3R000915BF.html
http://yuedu.163.com/book_reader/b39efe40b81843a8ac4eabdd3b756d92_4/cd59ff87a38e48eba21b312c4d26f2c7_4?utm_campaign=163ad&utm_source=163home&utm_medium=tab_1_2_7
http://v.163.com/special/opencourse/financialmarkets.html
http://paopao.163.com/schedule/show?pageId=4050&utm_source=163&utm_medium=wytab01&utm_campaign=warmup
http://xf.house.163.com/zz/search/0-0-0-0-0-0-0-0-1.html
http://sports.163.com/15/0321/10/AL7MA69F00052UUC.html
http://ent.163.com/15/0321/01/AL6NG0GI00031H2L.html
http://img2.cache.netease.com/lady/2014/3/1/201403012352473e66b.jpg
http://love.163.com/?vendor=163.navi.icon&utm_source=163.com&utm_campaign=163navi
http://caipiao.163.com/#from=www
http://money.163.com/15/0321/08/AL7GDD1L00253B0H.html
http://yichuangqingshu.lofter.com/post/21d053_641bd4b?act=qbwysylofer_20150101_01
http://img4.cache.netease.com/tech/2015/3/21/20150321095714dd3c3.jpg
http://m.163.com/iphone/index.html
http://yuanst.blog.163.com/blog/static/186229043201522084612809/
http://lady.163.com/15/0320/00/AL42J3UD00264OCL.html
http://w.163.com/15/0320/15/AL5MBP6J00314C3U.html
http://vhouse.163.com/1421889369882.html
http://img2.cache.netease.com/edu/2015/3/20/2015032017293274fa5.jpg
posted @
2015-03-21 16:59 marchalex 阅读(413) |
评论 (0) |
编辑 收藏
今天开始准备写一个Java可以跑得python编译器。
基本假设是这样的:
有一个JFrame,里面有一框,是输入框也是输出框,一开始如果有需要输入的内容得先写到上面去;
然后单击菜单栏打开python文件;
然后得到的结果就会显示出来。
目前知识有了一个框架,当test.py内容为:
print 123456789+123456789000000000
时,运行程序,现实的效果如下:
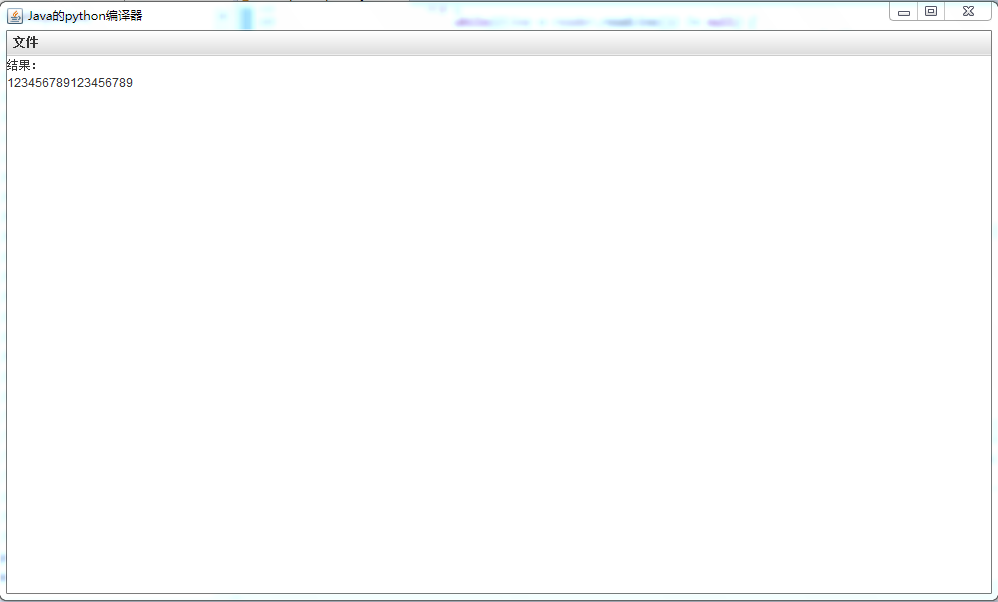
代码如下:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.StringTokenizer;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JTextArea;
public class CompilerOpener extends JFrame {
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static JTextArea textArea = null;
public CompilerOpener() {
frame = new JFrame("Java的python编译器");
textArea = new JTextArea();
textArea.setLineWrap(true);// 激活自动换行功能
frame.add(textArea);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("文件");
JMenuItem openItem = new JMenuItem("打开");
openItem.addActionListener(new MyAction());
fileMenu.add(openItem);
menuBar.add(fileMenu);
frame.setLocationRelativeTo(null);
frame.setSize(Width, Height);
frame.setLocation(100, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
private class MyAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
//Object s = evt.getSource();
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "选择");
File file=jfc.getSelectedFile();
int file_len = file.toString().length();
String ans = new String();
if(!file.isFile() || !file.toString().substring(file_len-3, file_len).equals(".py")) {
ans = "请确定你选择的文件是一个正确的python文件!";
} else {
String content = textArea.getText();
ans += content;
ans += "结果:\n";
try {
BufferedReader reader = new BufferedReader(new FileReader(file.toString()));
String line = null;
try {
while((line = reader.readLine()) != null) {
StringTokenizer st = new StringTokenizer(line , " +");
String s , a , b;
s = st.nextToken();
a = st.nextToken();
b = st.nextToken();
char[] ca = a.toCharArray();
char[] cb = b.toCharArray();
int lena = ca.length, lenb = cb.length;
int c = 0,len = lena > lenb ? lena : lenb;
int[] aa = new int[len+1];
for(int i=0;i<len;i++) {
if(lena-1-i >= 0)
c += (ca[lena-1-i] - '0');
if(lenb-1-i >= 0)
c += (cb[lenb-1-i] - '0');
aa[i] = c % 10;
c /= 10;
}
if(c > 0) aa[len++] = 1;
for(int i=len-1;i>=0;i--) ans += aa[i];
ans += "\n";
}
textArea.setText(ans);
} catch (IOException e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
new CompilerOpener();
}
}
posted @
2015-03-20 14:19 marchalex 阅读(188) |
评论 (0) |
编辑 收藏

代码如下:
import java.util.Scanner;
public class Perceptron {
private static int N = 3;
private static int n = 2;
private static double[][] X = null;
private static double[] Y = null;
private static double[][] G = null;
private static double[] A = null;
private static double[] W = null;
private static double B = 0;
private static double fi = 0.5;
private static boolean check(int id) {
double ans = B;
for(int i=0;i<N;i++)
ans += A[i] * Y[i] * G[i][id];
if(ans * Y[id] > 0) return true;
return false;
}
public static void solve() {
Scanner in = new Scanner(System.in);
System.out.print("input N:"); N = in.nextInt();
System.out.print("input n:"); n = in.nextInt();
X = new double[N][n];
Y = new double[N];
G = new double[N][N];
System.out.println("input N * n datas X[i][j]:");
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
X[i][j] = in.nextDouble();
System.out.println("input N datas Y[i]");
for(int i=0;i<N;i++)
Y[i] = in.nextDouble();
for(int i=0;i<N;i++)
for(int j=0;j<N;j++) {
G[i][j] = 0;
for(int k=0;k<n;k++)
G[i][j] += X[i][k] * X[j][k];
}
A = new double[N];
W = new double[n];
for(int i=0;i<n;i++) A[i] = 0;
B = 0;
boolean ok = true;
while(ok == true) {
ok = false;
//这里在原来算法的基础上不断地将fi缩小,以避免跳来跳去一直达不到要求的点的效果。
for(int i=0;i<N;i++) {
//System.out.println("here " + i);
while(check(i) == false) {
ok = true;
A[i] += fi;
B += fi * Y[i];
//debug();
}
}
fi *= 0.5;
}
for(int i=0;i<n;i++)
W[i] = 0;
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
W[j] += A[i] * Y[i] * X[i][j];
}
public static void main(String[] args) {
solve();
System.out.print("W = [");
for(int i=0;i<n-1;i++) System.out.print(W[i] + ", ");
System.out.println(W[n-1] + "]");
System.out.println("B = " + B);
}
}
posted @
2015-03-20 11:34 marchalex 阅读(871) |
评论 (0) |
编辑 收藏

实现时遇到一个问题,就是fi的值的设定问题,因为我们采用随机梯度下降法,一方面这节省了时间,但是如果fi值亘古不变的话可能会面临跳来跳去一直找不到答案的问题,所以我这里设定他得知在每一轮之后都会按比例减小(fi *= 0.5;),大家可以按自己的喜好自由设定。
import java.util.Scanner;
public class Perceptron {
private static int N = 3;
private static int n = 2;
private static double[][] X = null;
private static double[] Y = null;
private static double[] W = null;
private static double B = 0;
private static double fi = 0.5;
private static boolean check(int id) {
double ans = B;
for(int i=0;i<n;i++)
ans += X[id][i] * W[i];
if(ans * Y[id] > 0) return true;
return false;
}
private static void debug() {
System.out.print("debug: W");
for(int i=0;i<n;i++) System.out.print(W[i] + " ");
System.out.println("/ B : " + B);
}
public static void solve() {
Scanner in = new Scanner(System.in);
System.out.print("input N:"); N = in.nextInt();
System.out.print("input n:"); n = in.nextInt();
X = new double[N][n];
Y = new double[N];
W = new double[n];
System.out.println("input N * n datas X[i][j]:");
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
X[i][j] = in.nextDouble();
System.out.println("input N datas Y[i]");
for(int i=0;i<N;i++)
Y[i] = in.nextDouble();
for(int i=0;i<n;i++) W[i] = 0;
B = 0;
boolean ok = true;
while(ok == true) {
ok = false;
//这里在原来算法的基础上不断地将fi缩小,以避免跳来跳去一直达不到要求的点的效果。
for(int i=0;i<N;i++) {
//System.out.println("here " + i);
while(check(i) == false) {
ok = true;
for(int j=0;j<n;j++)
W[j] += fi * Y[i] * X[i][j];
B += fi * Y[i];
//debug();
}
}
fi *= 0.5;
}
}
public static void main(String[] args) {
solve();
System.out.print("W = [");
for(int i=0;i<n-1;i++) System.out.print(W[i] + ", ");
System.out.println(W[n-1] + "]");
System.out.println("B = " + B);
}
}
posted @
2015-03-20 11:08 marchalex 阅读(641) |
评论 (0) |
编辑 收藏
这个应用主要是为了生成座位的安排。程序运行后,在菜单栏中选择打开文件,然后我们假设文件的格式为:每一行一个人名。
例:
风清扬
无名僧
东方不败
任我行
乔峰
虚竹
段誉
杨过
郭靖
黄蓉
周伯通
小龙女
这个程序的特点如下:
在JFrame中添加了菜单栏;
在画布中添加了诸多元素;
最后会将图片通过图片缓存保存到本地“frame.png”中。
代码如下:
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
public class FrameWork extends JFrame {
private static final double pi = Math.acos(-1.0);
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static int personNumber = 15;
private static String[] names = new String[100];
public FrameWork() {
frame = new JFrame("Java菜单栏");
//JList list = new JList();
//frame.add(list);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("文件");
JMenu openMenu = new JMenu("打开");
JMenuItem openItem = new JMenuItem("文件");
openMenu.add(openItem);
openItem.addActionListener(new MyAction());
fileMenu.add(openMenu);
menuBar.add(fileMenu);
frame.setLocationRelativeTo(null);
frame.setSize(Width, Height);
frame.setLocation(100, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//JPanel panel = new ImagePanel();
//panel.setBounds(0, 0, Width, Height);
//frame.getContentPane().add(panel);
frame.setVisible(true);
}
private static int getNumber1(int i) {
int n = personNumber;
if((n-1)/2*2-2*i >= 0) return (n-1)/2*2 - 2*i;
return (i-(n-1)/2)*2-1;
}
private static int getNumber2(int i) {
if(i*2+1 <= personNumber) return i*2;
return 2*(personNumber-i)-1;
}
private class MyAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
//Object s = evt.getSource();
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "选择");
File file=jfc.getSelectedFile();
/*if(file.isDirectory()){
System.out.println("文件夹:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}*/
personNumber = 0;
BufferedReader reader;
try {
reader = new BufferedReader(new FileReader(file.getAbsolutePath()));
while((names[personNumber]=reader.readLine()) != null) {
personNumber ++;
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, Width, Height);
frame.getContentPane().add(panel);
frame.setVisible(true);
//System.out.println(personNumber);
//for(int i=0;i<personNumber;i++)
//System.out.println(names[i]);
BufferedImage bi = new BufferedImage(frame.getWidth(), frame.getHeight(), BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = bi.createGraphics();
frame.paint(g2d);
try {
ImageIO.write(bi, "PNG", new File("D:\\frame.png"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.white);
g.fillRect(0, 0, Width, Height);
g.setColor(Color.black);
int delx = (Width - 20) / (personNumber + 1);
for(int i=0;i<personNumber;i++) {
int x = i * delx + 10;
g.drawRect(x, 10, delx, 20);
//String s = "" + getNumber1(i);
int id = getNumber1(i);
String s = names[id];
g.drawString(s, x+2, 22);
//s = "" + getNumber2(i);
id = getNumber2(i);
s = names[id];
int x0 = 440, y0 = 285, r = 170;
int xx = (int) (x0 - r * Math.sin(pi*2*i/personNumber));
int yy = (int) (y0 - r * Math.cos(pi*2*i/personNumber));
g.drawString(s, xx, yy);
}
g.drawOval(300, 130, 300, 300);
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-03-18 20:20 marchalex 阅读(293) |
评论 (0) |
编辑 收藏
写了一个FrameWork类实现了菜单栏,并且为菜单栏里的Item添加事件监听,实现了选择文件的功能。
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
public class FrameWork extends JFrame {
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
public FrameWork() {
frame = new JFrame("Java菜单栏");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("文件");
JMenu openMenu = new JMenu("打开");
JMenuItem openItem = new JMenuItem("文件");
openMenu.add(openItem);
openItem.addActionListener(new MyAction());
fileMenu.add(openMenu);
menuBar.add(fileMenu);
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setLocation(100, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class MyAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
Object s = evt.getSource();
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "选择");
File file=jfc.getSelectedFile();
if(file.isDirectory()){
System.out.println("文件夹:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}
System.out.println(jfc.getSelectedFile().getName());
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-03-18 12:51 marchalex 阅读(953) |
评论 (0) |
编辑 收藏
setFileSelectionMode(int mode)
设置 JFileChooser
,以允许用户只选择文件、只选择目录,或者可选择文件和目录。
mode参数:FILES_AND_DIRECTORIES
指示显示文件和目录。
FILES_ONLY
指示仅显示文件。
DIRECTORIES_ONLY
指示仅显示目录。
showDialog(Component parent,String approveButtonText)
弹出具有自定义 approve 按钮的自定义文件选择器对话框。
showOpenDialog(Component parent)
弹出一个 "Open File" 文件选择器对话框。
showSaveDialog(Component parent)
弹出一个 "Save File" 文件选择器对话框。
setMultiSelectionEnabled(boolean b)
设置文件选择器,以允许选择多个文件。
getSelectedFiles()
如果将文件选择器设置为允许选择多个文件,则返回选中文件的列表(File[])。
getSelectedFile()
返回选中的文件。
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class FileChooser extends JFrame implements ActionListener{
JButton open=null;
public static void main(String[] args) {
new FileChooser();
}
public FileChooser(){
open=new JButton("open");
this.add(open);
this.setBounds(400, 200, 100, 100);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
open.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "选择");
File file=jfc.getSelectedFile();
if(file.isDirectory()){
System.out.println("文件夹:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}
System.out.println(jfc.getSelectedFile().getName());
}
}
posted @
2015-03-18 12:35 marchalex 阅读(577) |
评论 (0) |
编辑 收藏
今天写了一个在JFrame显示图片(包括动图)的小程序。
主要用到了
JPanel类,JPanel类有一个paint()方法,用于实现画图。在这里paint()方法里写的就是调用一张图片,然后就实现了在JFrame中显示一张图片。
其原理其实是:在JFrame对象中放一个JPanel对象,在JPanel中实现画图。
代码如下:
import java.awt.Graphics;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class ImageApp extends JFrame {
public ImageApp() {
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setSize(400, 300);
setResizable(false);
getContentPane().setLayout(null);
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, 400, 300);
getContentPane().add(panel);
setVisible(true);
}
public static void main(String[] args) {
new ImageApp();
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
ImageIcon icon = new ImageIcon("D:\\testapp.jpg");
g.drawImage(icon.getImage(), 0, 0, 400, 300, this);
}
}
}
动图如下:(D:\\testapp.jpg)
posted @
2015-03-13 11:32 marchalex 阅读(3311) |
评论 (0) |
编辑 收藏
在之前写过一个
TranslateHelper类用于在线翻译英文单词。
我之前写过一个
单词翻译大师用于将文件中出现的所有单词存储在另一个文件中。存储的格式如下:
word: explain
如:
apple: 苹果;苹果树;苹果公司
banana: 香蕉;芭蕉属植物;喜剧演员
我们假设这里获取单词的原文件为D:|test_english.txt
存储单词的文件为D:\word_lib.txt
这次写的TranslateHelper2类就是在此基础上编写的一个英汉词典的离线版本。
在此之前我写了一个WordFinder类用于获取D:\word_lib.txt下的特定单词及其解释(没有的话返回null)。
WordFinder.java
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.StringTokenizer;
public class WordFinder {
public static String find(String word) throws Exception {
String filename = new String("D:\\word_lib.txt");
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line = "";
while((line = reader.readLine()) != null) {
StringTokenizer st = new StringTokenizer(line, ":");
String key = st.nextToken();
if(key.equals(word)) {
return st.nextToken();
}
}
return null;
}
public static void main(String[] args) throws Exception {
String ans = find("apple");
System.out.println(ans);
}
}
下面是TranslateHelper2类,其词库是基于文件D:\word_lib.txt的,如下:
新增了一个按键可在线更新词库,即D:\word_lib.txt里面的内容(在现实点按键可更新)。
TranslateHelper2.java
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.net.HttpURLConnection;
import java.net.URL;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class TranslateHelper2 extends JFrame {
private static final int Width = 500;
private static final int Height = 220;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
private static JLabel label = null;
private static JTextField wordText = null;
private static JTextField explainText = null;
private static JButton button = null;
private static JButton new_button = null;
public TranslateHelper2() {
frame = new JFrame("Translate Helper");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
label = new JLabel("单词:");
wordText = new JTextField(10);
explainText = new JTextField(40);
button = new JButton("提交");
new_button = new JButton("在线时点击可更新");
frame.add(label);
frame.add(wordText);
frame.add(button);
frame.add(explainText);
frame.add(new_button);
button.addActionListener(new ButtonAction());
new_button.addActionListener(new ButtonAction());
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class ButtonAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
Object s = evt.getSource();
//System.out.println("hello");
if(s == button) {
String word = wordText.getText();
try {
String _word = word;
String _explain = WordFinder.find(word);
wordText.setText(_word);
explainText.setText(_explain);
} catch (Exception e) {
e.printStackTrace();
}
} else if(s == new_button) {
try {
TranslateMaster.translateAllLocal("D:\\test_english.txt", "D:\\word_lib.txt");
} catch (Exception e) {
return;
}
}
}
}
public static void main(String[] args) {
new TranslateHelper2();
}
}
posted @
2015-03-11 13:25 marchalex 阅读(417) |
评论 (0) |
编辑 收藏
之前写过
FileHelper类,其中的readFile和writeFile方法分别用于文件的读和写。这次在原来的基础上添加了如下方法:
- listFiles
- hasWords
- findFilesContainsWords
- 递归地查找某一目录下所有包含某一关键字的所有文件(这里我加了一个过滤器,即我只找了所有后缀为“.h”的文件)。
加了新功能后的FileHelper类代码如下:
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
public class FileHelper {
public static String readFile(String filename) throws Exception {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String ans = "", line = null;
while((line = reader.readLine()) != null){
ans += line + "\r\n";
}
reader.close();
return ans;
}
public static void writeFile(String content, String filename) throws Exception {
BufferedWriter writer = new BufferedWriter(new FileWriter(filename));
writer.write(content);
writer.flush();
writer.close();
}
public static void listFiles(String path) {
File file = new File(path);
File[] files = file.listFiles();
if(files == null)
return;
for(File f : files) {
if(f.isFile()) {
System.out.println(f.toString());
} else if(f.isDirectory()) {
System.out.println(f.toString());
listFiles(f.toString());
}
}
}
public static boolean hasWords(String file, String words) {
try {
String s = readFile(file);
int w_len = words.length();
int len = s.length();
for(int i=0;i+w_len<=len;i++) {
if(s.substring(i, i+w_len).equals(words))
return true;
}
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
public static void findFilesContainsWords(String path, String words) throws Exception {
File file = new File(path);
File[] files = file.listFiles();
if(files == null) return;
for(File f : files) {
if(f.isFile()) {
String s = f.toString();
int s_len = s.length();
if(s.substring(s_len-2, s_len).equals(".h") == false) continue; // add filter
if(hasWords(f.toString(), words))
System.out.println(f.toString());
} else if(f.isDirectory()) {
findFilesContainsWords(f.toString(), words);
}
}
}
public static void main(String[] args) throws Exception {
//String ans = readFile("D:\\input.txt");
//System.out.println(ans);
//writeFile(ans, "D:\\output.txt");
//findFilesContainsWords("D:\\clamav-0.98.6", "scanmanager");//在IDE中找
if(args.length != 1) {
System.out.println("Usage : \"D:\\clamav-0.98.6\" words");
return;
}
findFilesContainsWords("D:\\clamav-0.98.6", args[0]);//在命令行中找
}
}
posted @
2015-03-10 16:11 marchalex 阅读(598) |
评论 (0) |
编辑 收藏
这是我很多月以前学习
马士兵的Java教学视频的时候在教程的基础上稍微改进了一点的一个聊天室软件。
聊天室软件分为ChatClient类和ChatServer类。
ChatServer相当于一个服务器。
ChatClient类相当于一个客户端。
用户可以通过客户端进行聊天。
客户端登录时会询问你的姓名,输入姓名后大家就可以基于此聊天室软件进行聊天了。
CHatClient.java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.net.*;
public class ChatClient extends Frame {
Socket socket = null;
DataOutputStream dos = null;
DataInputStream dis = null;
private boolean bConnected = false;
private String name;
//private boolean named = false;
TextField tfTxt = new TextField();
TextArea taContent = new TextArea();
Thread tRecv = new Thread(new RecvThread());
public static void main(String[] args) {
new ChatClient().launchFrame();
}
public void launchFrame() {
setLocation(400, 300);
this.setSize(300, 300);
add(tfTxt, BorderLayout.SOUTH);
add(taContent, BorderLayout.NORTH);
pack();
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
disconnect();
System.exit(0);
}
});
setVisible(true);
connect();
tfTxt.addActionListener(new TFListener());
//new Thread(new RecvThread()).start();
tRecv.start();
}
public void connect() {
try {
socket = new Socket("127.0.0.1", 8888);
dos = new DataOutputStream(socket.getOutputStream());
dis = new DataInputStream(socket.getInputStream());
System.out.println("connected!");
taContent.setText("你叫什么名字?\n");
bConnected = true;
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public void disconnect() {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
/*
try {
bConnected = false;
tRecv.join();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
*/
}
private class TFListener implements ActionListener {
private boolean named = false;
public void actionPerformed(ActionEvent e) {
String str = tfTxt.getText().trim();
//taContent.setText(str);
tfTxt.setText("");
if(named == false) {
named = true;
name = str;
try {
dos.writeUTF(name+" is coming!");
dos.flush();
} catch (IOException e1) {
e1.printStackTrace();
}
return;
}
try {
//dos = new DataOutputStream(socket.getOutputStream());
dos.writeUTF(name + ":" + str);
dos.flush(); //dos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
private class RecvThread implements Runnable {
private String name;
public void run() {
/*
while(bConnected) {
try {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
*/
try {
while(bConnected) {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
}
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
ChatServer.java
import java.io.*;
import java.net.*;
import java.util.*;
public class ChatServer {
boolean started =
false;
ServerSocket ss =
null;
List<Client> clients =
new ArrayList<Client>();
public static void main(String[] args) {
new ChatServer().start();
}
public void start() {
try {
ss =
new ServerSocket(8888);
started =
true;
}
catch (BindException e) {
System.out.println("端口使用中

");
System.out.println("请关掉相应程序并重新运行服务器!");
System.exit(0);
}
catch (IOException e) {
e.printStackTrace();
}
try {
while(started) {
Socket s = ss.accept();
Client c =
new Client(s);
System.out.println("a client connected!");
new Thread(c).start();
clients.add(c);
}
}
catch (IOException e) {
e.printStackTrace();
}
finally {
try {
ss.close();
}
catch (IOException e) {
e.printStackTrace();
}
}
}
class Client
implements Runnable {
private Socket s;
private DataInputStream dis =
null;
private DataOutputStream dos =
null;
private boolean bConnected =
false;
public Client(Socket s) {
this.s = s;
try {
dis =
new DataInputStream(s.getInputStream());
dos =
new DataOutputStream(s.getOutputStream());
bConnected =
true;
}
catch (IOException e) {
e.printStackTrace();
}
}
public void send(String str) {
try {
dos.writeUTF(str);
}
catch (IOException e) {
clients.remove(
this);
System.out.println("对方退出了!我从List里面去掉了!");
//e.printStackTrace();
}
}
public void run() {
try {
while(bConnected) {
String str = dis.readUTF();
System.out.println(str);
for(
int i=0;i<clients.size();i++) {
Client c = clients.get(i);
c.send(str);
}
/*
for(Iterator<Client> it = clients.iterator(); it.hasNext(); ) {
Client c = it.next();
c.send(str);
}
*/
/*
Iterator<Client> it = clients.iterator();
while(it.hasNext()) {
Client c = it.next();
c.send(str);
}
*/
}
}
catch (EOFException e) {
System.out.println("Client closed!");
}
catch (IOException e) {
e.printStackTrace();
}
finally {
try {
if(dis !=
null) dis.close();
if(dos !=
null) dos.close();
if(s !=
null) {
s.close();
s =
null;
}
}
catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
}
posted @
2015-03-09 08:39 marchalex 阅读(581) |
评论 (0) |
编辑 收藏
上次写了一个基于iciba的
英汉翻译,这次在原来的基础上加了一个窗口,实现了TranslateHelper类。
效果如下:
代码如下:
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class TranslateHelper extends JFrame {
private static final int Width = 500;
private static final int Height = 200;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
private static JLabel label = null;
private static JTextField wordText = null;
private static JTextField explainText = null;
private static JButton button = null;
public TranslateHelper() {
frame = new JFrame("Translate Helper");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
label = new JLabel("单词:");
wordText = new JTextField(10);
explainText = new JTextField(40);
button = new JButton("提交");
frame.add(label);
frame.add(wordText);
frame.add(button);
frame.add(explainText);
button.addActionListener(new ButtonAction());
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class ButtonAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
//Object s = evt.getSource();
//System.out.println("hello");
String word = wordText.getText();
try {
String _word = EnglishChineseTranslater.getWordName(word);
String _explain = EnglishChineseTranslater.getTranslation(word);
wordText.setText(_word);
explainText.setText(_explain);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
new TranslateHelper();
}
}
posted @
2015-03-08 18:47 marchalex 阅读(212) |
评论 (0) |
编辑 收藏
MyFrame类用于实现数据的增删改查。
代码用JFrame进行了演示,其中的每一个按键都添加了事件监听。
这里假定mysql数据库的端口号是3306。
数据库是my_database。
表是user。
表中包含name和score两个元素。
使用前注意引入JDBC的jar包作为外部jar包。
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class MyFrame extends JFrame {
private static final int Width = 420;
private static final int Height = 300;
private static JFrame frame = null;
//private static Container ctn = null;
private static FlowLayout flowLayout = null;
private static JLabel nameLabel = null;
private static JLabel scoreLabel = null;
private static JTextField nameText = null;
private static JTextField scoreText = null;
private static JButton addButton = null;
private static JButton deleteButton = null;
private static JButton modifyButton = null;
private static JButton searchButton = null;
private static JLabel resultLabel = null;
private static JTextField resultText = null;
private static JButton closeButton = null;
private static String driver = "com.mysql.jdbc.Driver";
private static String url = "jdbc:mysql://localhost:3306/my_database";
private static String userName = "root";
private static String password = "root";
private static Connection conn = null;
private static Statement stmt = null;
public MyFrame() {
frame = new JFrame("JFrame connect MySQL");
flowLayout = new FlowLayout(FlowLayout.LEFT);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
nameLabel = new JLabel("name");
scoreLabel = new JLabel("score");
nameText = new JTextField(10);
scoreText = new JTextField(10);
addButton = new JButton("ADD");
deleteButton = new JButton("DELETE");
modifyButton = new JButton("MODIFY");
searchButton = new JButton("SEARCH");
resultLabel = new JLabel("result");
resultText = new JTextField(30);
closeButton = new JButton("CLOSE");
frame.add(nameLabel);
frame.add(nameText);
frame.add(scoreLabel);
frame.add(scoreText);
frame.add(addButton);
frame.add(deleteButton);
frame.add(modifyButton);
frame.add(searchButton);
frame.add(resultLabel);
frame.add(resultText);
frame.add(closeButton);
addButton.addActionListener(new ButtonAction());
deleteButton.addActionListener(new ButtonAction());
modifyButton.addActionListener(new ButtonAction());
searchButton.addActionListener(new ButtonAction());
closeButton.addActionListener(new ButtonAction());
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class ButtonAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
Object s = evt.getSource();
String name = nameText.getText();
String score = scoreText.getText();
if(s == addButton) {
if(name.length()==0 || score.length()==0) {
System.out.println("Please enter the name and score!");
}
else {
String sqlInsert =
"INSERT INTO my_table (name,score) VALUES (\"" + name + "\"," + score + ");";
try {
stmt.executeUpdate(sqlInsert);
} catch (SQLException e) {
e.printStackTrace();
}
System.out.println("Add " + name + " Succeed!");
}
} else if(s == deleteButton) {
if(name.length() == 0) {
System.out.println("You must enter the name");
return;
} else if(score.length() == 0) {
String sqlDelete = "DELETE FROM my_table WHERE name=\"" + name + "\";";
try {
stmt.executeUpdate(sqlDelete);
} catch (SQLException e) {
e.printStackTrace();
}
} else {
String sqlDelete = "DELETE FROM my_table WHERE name=\"" + name
+ "\" and score<=" + score + ";";
try {
stmt.executeUpdate(sqlDelete);
} catch (SQLException e) {
e.printStackTrace();
}
}
System.out.println("Delete succeed!");
} else if(s == modifyButton) {
if(name.length() == 0 || score.length() == 0) {
System.out.println("Please enter the name and score you want to modify!");
} else {
String sqlModify = "UPDATE my_table SET name=\"" + name
+ "\" WHERE score=" + score + ";";
try {
stmt.executeUpdate(sqlModify);
} catch (SQLException e) {
e.printStackTrace();
}
System.out.println("Modify " + name + " succeed!");
}
} else if(s == searchButton) {
String sqlName = " name=\"" + name + "\"";
String sqlScore = " score=" + score;
String sqlSelect = "SELECT * FROM my_table";
if(name.length() == 0 && score.length() == 0) {
;
} else if(score.length() == 0) {
sqlSelect += " WHERE" + sqlName + ";";
} else if(name.length() == 0) {
sqlSelect += " WHERE" + sqlScore + ";";
} else {
sqlSelect += " WHERE" + sqlName + " and" + sqlScore + ";";
}
//System.out.println(sqlSelect);
try {
ResultSet res = stmt.executeQuery(sqlSelect);
while(res.next()) {
String ansName = res.getString(1);
String ansScore = res.getString(2);
System.out.println(ansName + " get score: " + ansScore);
}
} catch (SQLException e) {
e.printStackTrace();
}
} else if(s == closeButton) {
try {
stmt.close();
if(conn != null)
conn.close();
} catch (SQLException e) {
System.out.println("SQLException异常"+e.getMessage());
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
try {
Class.forName(driver);
System.out.println("数据库连接成功");
} catch (ClassNotFoundException e) {
System.out.println("ClassNotFoundException");
}
try {
conn = DriverManager.getConnection(url, userName, password);
System.out.println("connect database successful");
stmt = conn.createStatement();
new MyFrame();
} catch (SQLException e) {
System.out.println("SQLException异常"+e.getMessage());
e.printStackTrace();
}
}
}
posted @
2015-03-08 16:43 marchalex 阅读(668) |
评论 (0) |
编辑 收藏
MapReduce是一种可用于数据处理的变成模型。
这里主要讲述Java语言实现MapReduce。
一个投票模型:
Jobs很喜欢给女生打分,好看的打100分,难看的打0分。有一次他给Lucy打了0分,结果被Lucy痛打了一顿。
还有一次,Jobs给两个美女打分,给美女Alice打了99分,给美女Candice打了98分。
这个时候Alex就看不下去了,他于是站起来说:“明明是Candice比较好看嘛!”。
两人于是争执起来,为了证明自己的观点,结果爆发了一场大战!什么降龙十八掌啊,黯然销魂掌啊,他们都不会。
那么怎么才能让对方输的心服口服呢?他们想到“群众的眼睛是雪亮的”!于是他们发动了班上的20名同学进行投票。
结果出来了,Alice的平均分是98.5,Candice的平均分是99.7,以压倒性的优势获得了胜利。
但是Jobs不服,于是把班上每个女生的照片放到了网上,让大家来评分,最后他得到了好多文件,用自己的电脑算根本忙不过来,于是他想到了用Hadoop写一个MapReduce程序。
一直输入文件的格式是:"[user]\t[girlname]\t[point]".例:
alex alice 88
alex candice 100
jobs alice 100
john lucy 89
在这里,我们假设每个人的评分都为0到100的整数,最终的结果向下取整。那么MapReduce程序可以写成如下:
我们需要三样东西:一个map函数,一个reduce函数,和一些用来运行作业的代码。
map函数由Mapper接口实现来表示,后者声明了一个map()方法。
AverageMapper.java
import java.io.IOException;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapred.MapReduceBase;
import org.apache.hadoop.mapred.Mapper;
import org.apache.hadoop.mapred.OutputCollector;
import org.apache.hadoop.mapred.Reporter;
public class AverageMapper extends MapReduceBase
implements Mapper<IntWritable, Text, Text, IntWritable> {
public void map(IntWritable key, Text value,
OutputCollector<Text, IntWritable> output, Reporter reporter)
throws IOException {
String s = value.toString();
String name = new String();
int point = 0;
int i;
for(i=0;i<s.length() && s.charAt(i)!='\t';i++);
for(i++;i<s.length() && s.charAt(i)!='\t';i++) {
name += s.charAt(i);
}
for(i++;i<s.length();i++) {
point = point * 10 + (s.charAt(i) - '0');
}
if(name.length() != 0 && point >=0 && point <= 100) {
output.collect(new Text(name), new IntWritable(point));
}
}
}
该Mapper接口是一个泛型类型,他有四个形参类型,分别指定map函数的输入键、输入值、输出键、输出值的类型。
以示例来说,输入键是一个整形偏移量(表示行号),输入值是一行文本,输出键是美女的姓名,输出值是美女的得分。
Hadoop自身提供一套可优化网络序列化传输的基本类型,而不直接使用Java内嵌的类型。这些类型均可在org.apache.hadoop.io包中找到。
这里我们使用IntWritable类型(相当于Java中的Integer类型)和Text类型(想到与Java中的String类型)。
map()方法还提供了OutputCollector示例用于输出内容的写入。
我们只在输入内容格式正确的时候才将其写入输出记录中。
reduce函数通过Reducer进行类似的定义。
AverageReducer.java
import java.io.IOException;
import java.util.Iterator;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapred.MapReduceBase;
import org.apache.hadoop.mapred.OutputCollector;
import org.apache.hadoop.mapred.Reducer;
import org.apache.hadoop.mapred.Reporter;
public class AverageReducer extends MapReduceBase
implements Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterator<IntWritable> values,
OutputCollector<Text, IntWritable> output, Reporter reporter)
throws IOException {
long tot_point = 0, num = 0;
while(values.hasNext()) {
tot_point += values.next().get();
num ++;
}
int ave_point = (int)(tot_point/num);
output.collect(key, new IntWritable(ave_point));
}
}
第三部分代码负责运行MapReduce作业。
Average.java
import java.io.IOException;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapred.FileInputFormat;
import org.apache.hadoop.mapred.FileOutputFormat;
import org.apache.hadoop.mapred.JobConf;
public class Average {
public static void main(String[] args) throws IOException {
if(args.length != 2) {
System.err.println("Usage: Average <input path> <output path>");
System.exit(-1);
}
JobConf conf = new JobConf(Average.class);
conf.setJobName("Average");
FileInputFormat.addInputPath(conf, new Path(args[0]));
FileOutputFormat.setOutputPath(conf, new Path(args[1]));
conf.setMapperClass(AverageMapper.class);
conf.setReducerClass(AverageReducer.class);
conf.setOutputKeyClass(Text.class);
conf.setOutputValueClass(IntWritable.class);
}
}
JobConf对象指定了作业执行规范。我们可以用它来控制整个作业的运行。
在Hadoop作业上运行着写作业时,我们需要将代码打包成一个JAR文件(Hadoop会在集群上分发这些文件)。
我们无需明确指定JAR文件的名称,而只需在JobConf的构造函数中传递一个类,Hadoop将通过该类查找JAR文件进而找到相关的JAR文件。
构造JobCOnf对象之后,需要指定输入和输出数据的路径。
调用FileInputFormat类的静态方法addInputPath()来定义输入数据的路径。
可以多次调用addInputOath()实现多路径的输入。
调用FileOutputFormat类的静态函数SetOutputPath()来指定输出路径。
该函数指定了reduce函数输出文件的写入目录。
在运行任务前该目录不应该存在,否则Hadoop会报错并拒绝运行该任务。
这种预防措施是为了防止数据丢失。
接着,通过SetMapperClass()和SetReducerClass()指定map和reduce类型。
输入类型通过InputFormat类来控制,我们的例子中没有设置,因为使用的是默认的TextInputFormat(文本输入格式)。
新增的Java MapReduce API
新的Hadoop在版本0.20.0包含有一个新的Java MapReduce API,又是也称为"上下文对象"(context object),旨在使API在今后更容易扩展。
新特性:
倾向于使用虚类,而不是接口;
新的API放在org.apache.hadoop.mapreduce包中(旧版本org.apache.hadoop.mapred包);
新的API充分使用上下文对象,使用户代码能与MapReduce通信。例如:MapContext基本具备了JobConf,OutputCollector和Reporter的功能;
新的API同时支持“推”(push)和“拉”(pull)的迭代。这两类API,均可以将键/值对记录推给mapper,但除此之外,新的API也允许把记录从map()方法中拉出、对reducer来说是一样的。拉式处理的好处是可以实现批量处理,而非逐条记录的处理。
新的API实现了配置的统一。所有作业的配置均通过Configuration来完成。(区别于旧API的JobConf)。
新API中作业控制由Job类实现,而非JobClient类,新API中删除了JobClient类。
输出文件的命名稍有不同。
用新上下文对象来重写Average应用
import java.io.IOException;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class NewAverage {
static class NewAverageMapper
extends Mapper<IntWritable, Text, Text, IntWritable> {
public void map(IntWritable key, Text value, Context context)
throws IOException, InterruptedException {
String s = value.toString();
String name = new String();
int point = 0;
int i;
for(i=0;i<s.length() && s.charAt(i)!='\t';i++);
for(i++;i<s.length() && s.charAt(i)!='\t';i++) {
name += s.charAt(i);
}
for(i++;i<s.length();i++) {
point = point * 10 + (s.charAt(i) - '0');
}
if(name.length() != 0 && point >=0 && point <= 100) {
context.write(new Text(name), new IntWritable(point));
}
}
}
static class NewAverageReducer
extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values,
Context context)
throws IOException, InterruptedException {
long tot_point = 0, num = 0;
for(IntWritable value : values) {
tot_point += value.get();
num ++;
}
int ave_point = (int)(tot_point/num);
context.write(key, new IntWritable(ave_point));
}
}
public static void main(String[] args) throws Exception {
if(args.length != 2) {
System.err.println("Usage: NewAverage <input path> <output path>");
System.exit(-1);
}
Job job = new Job();
job.setJarByClass(NewAverage.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
job.setMapperClass(NewAverageMapper.class);
job.setReducerClass(NewAverageReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
posted @
2015-03-08 13:27 marchalex 阅读(1396) |
评论 (0) |
编辑 收藏
之前我写过获得tmall上qfour某单品页上第一张图片的
程序。
这次我又在qfour上面选了一个
猫娘志2015春秋新款女装显瘦小清新碎花长袖田园连衣裙女单品页用于获得下面的这张图。

这张图对应的url为:
http://gi4.md.alicdn.com/bao/uploaded/i4/TB1aRpnHpXXXXaNXVXXXXXXXXXX_!!0-item_pic.jpg
我写了一个ImageDownload类,其中的download方法用于下载url对应的图片并保存在特定的本地目录上。代码如下:
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
public class ImageDownload {
public static void download(String urlString, String filename) throws Exception {
URL url = new URL(urlString); // 构造URL
URLConnection con = url.openConnection(); // 打开链接
con.setConnectTimeout(5*1000); //设置请求超时为5s
InputStream is = con.getInputStream(); // 输入流
byte[] bs = new byte[1024]; // 1K的数据缓冲
int len; // 读取到的数据长度
int i = filename.length();
for(i--;i>=0 && filename.charAt(i) != '\\' && filename.charAt(i) != '/';i--);
String s_dir = filename.substring(0, i);
File dir = new File(s_dir); // 输出的文件流
if(!dir.exists()){
dir.mkdirs();
}
OutputStream os = new FileOutputStream(filename);
// 开始读取
while ((len = is.read(bs)) != -1) {
os.write(bs, 0, len);
}
// 完毕,关闭所有链接
os.close();
is.close();
}
public static void main(String[] args) throws Exception {
download("http://gi4.md.alicdn.com/bao/uploaded/i4/TB1aRpnHpXXXXaNXVXXXXXXXXXX_!!0-item_pic.jpg", "d:\\qfour\\sample.jpg");
}
}
posted @
2015-03-08 12:33 marchalex 阅读(1768) |
评论 (0) |
编辑 收藏
检测设备的运行状态,有的是使用ping的方式来检测的。所以需要使用java来实现ping功能。
为了使用java来实现ping的功能,有人推荐使用java的 Runtime.exec()方法来直接调用系统的Ping命令,也有人完成了纯Java实现Ping的程序,使用的是Java的NIO包(native io, 高效IO包)。但是设备检测只是想测试一个远程主机是否可用。所以,可以使用以下三种方式来实现:
1.Jdk1.5的InetAddresss方式
自从Java 1.5,java.net包中就实现了ICMP ping的功能。
见:Ping类的ping(String)函数。
使用时应注意,如果远程服务器设置了防火墙或相关的配制,可能会影响到结果。另外,由于发送ICMP请求需要程序对系统有一定的权限,当这个权限无法满足时, isReachable方法将试着连接远程主机的TCP端口 7(Echo)。
2.最简单的办法,直接调用CMD
见Ping类的ping02(String)函数。
3.Java调用控制台执行ping命令
具体的思路是这样的:
通过程序调用类似“ping 127.0.0.1 -n 10 -w 4”的命令,这命令会执行ping十次,如果通顺则会输出类似“来自127.0.0.1的回复: 字节=32 时间<1ms TTL=64”的文本(具体数字根据实际情况会有变化),其中中文是根据环境本地化的,有些机器上的中文部分是英文,但不论是中英文环境, 后面的“<1ms TTL=62”字样总是固定的,它表明一次ping的结果是能通的。如果这个字样出现的次数等于10次即测试的次数,则说明127.0.0.1是百分之百能连通的。
技术上:具体调用dos命令用Runtime.getRuntime().exec实现,查看字符串是否符合格式用正则表达式实现。
见Ping类的ping(String,int,int)函数。
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Ping {
public static boolean ping(String ipAddress) throws Exception {
int timeOut = 3000 ; //超时应该在3钞以上
boolean status = InetAddress.getByName(ipAddress).isReachable(timeOut); // 当返回值是true时,说明host是可用的,false则不可。
return status;
}
public static void ping02(String ipAddress) throws Exception {
String line = null;
try {
Process pro = Runtime.getRuntime().exec("ping " + ipAddress);
BufferedReader buf = new BufferedReader(new InputStreamReader(
pro.getInputStream()));
while ((line = buf.readLine()) != null)
System.out.println(line);
} catch (Exception ex) {
System.out.println(ex.getMessage());
}
}
public static boolean ping(String ipAddress, int pingTimes, int timeOut) {
BufferedReader in = null;
Runtime r = Runtime.getRuntime(); // 将要执行的ping命令,此命令是windows格式的命令
String pingCommand = "ping " + ipAddress + " -n " + pingTimes + " -w " + timeOut;
try { // 执行命令并获取输出
System.out.println(pingCommand);
Process p = r.exec(pingCommand);
if (p == null) {
return false;
}
in = new BufferedReader(new InputStreamReader(p.getInputStream())); // 逐行检查输出,计算类似出现=23ms TTL=62字样的次数
int connectedCount = 0;
String line = null;
while ((line = in.readLine()) != null) {
connectedCount += getCheckResult(line);
} // 如果出现类似=23ms TTL=62这样的字样,出现的次数=测试次数则返回真
return connectedCount == pingTimes;
} catch (Exception ex) {
ex.printStackTrace(); // 出现异常则返回假
return false;
} finally {
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//若line含有=18ms TTL=16字样,说明已经ping通,返回1,否則返回0.
private static int getCheckResult(String line) { // System.out.println("控制台输出的结果为:"+line);
Pattern pattern = Pattern.compile("(\\d+ms)(\\s+)(TTL=\\d+)", Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(line);
while (matcher.find()) {
return 1;
}
return 0;
}
public static void main(String[] args) throws Exception {
String ipAddress = "127.0.0.1";
System.out.println(ping(ipAddress));
ping02(ipAddress);
System.out.println(ping(ipAddress, 5, 5000));
}
}
小结:
第一种方法:Jdk1.5的InetAddresss,代码简单。
第二种方法:使用java调用cmd命令,这种方式最简单,可以把ping的过程显示在本地。
第三种方法:也是使用java调用控制台的ping命令,这个比较可靠,还通用,使用起来方便:传入个ip,设置ping的次数和超时,就可以根据返回值来判断是否ping通。
posted @
2015-03-08 09:48 marchalex 阅读(22170) |
评论 (3) |
编辑 收藏