为了防止某些用户使用软件进行登录和发布信息,很多网站在用户登录或者发布信息时,都要求用户输入验证码。验证码通常是以一幅图片的形式显示的,用户按照图片中显示的数字或者字母依次输入,服务端将对用户输入和验证码进行比较,以判断用户是否经过检验。由于验证码都是随机生成的,自动发布信息的软件无法知道生成的验证码。
1
生成图片的程序:
2
/**//*
3
* RandomCodeServlet.java
4
*
5
* Created on 2007-5-29, 0:51:02
6
*
7
* To change this template, choose Tools | Template Manager
8
* and open the template in the editor.
9
*/
10
11
package com.javahe.image;
12
13
/** *//**
14
*
15
* @author java-he
16
*/
17
import java.awt.Color;
18
import java.awt.Font;
19
import java.awt.Graphics2D;
20
import java.awt.image.BufferedImage;
21
import java.util.Random;
22
import javax.imageio.ImageIO;
23
24
import javax.servlet.*;
25
import java.io.*;
26
import javax.servlet.http.*;
27
28
public class RandomCodeServlet extends HttpServlet
{
29
//验证码图片的宽度。
30
private int width=60;
31
//验证码图片的高度。
32
private int height=20;
33
34
protected void service(HttpServletRequest req, HttpServletResponse resp)
35
throws ServletException, java.io.IOException
{
36
BufferedImage buffImg=new BufferedImage(width,height,
37
BufferedImage.TYPE_INT_RGB);
38
Graphics2D g=buffImg.createGraphics();
39
40
//创建一个随机数生成器类。
41
Random random=new Random();
42
43
g.setColor(Color.WHITE);
44
g.fillRect(0,0,width,height);
45
46
//创建字体,字体的大小应该根据图片的高度来定。
47
Font font=new Font("Times New Roman",Font.PLAIN,18);
48
//设置字体。
49
g.setFont(font);
50
51
//画边框。
52
g.setColor(Color.BLACK);
53
g.drawRect(0,0,width-1,height-1);
54
55
//随机产生160条干扰线,使图象中的认证码不易被其它程序探测到。
56
g.setColor(Color.GRAY);
57
for (int i=0;i<160;i++)
{
58
int x = random.nextInt(width);
59
int y = random.nextInt(height);
60
int xl = random.nextInt(12);
61
int yl = random.nextInt(12);
62
g.drawLine(x,y,x+xl,y+yl);
63
}
64
65
66
//randomCode用于保存随机产生的验证码,以便用户登录后进行验证。
67
StringBuffer randomCode=new StringBuffer();
68
int red=0,green=0,blue=0;
69
70
//随机产生4位数字的验证码。
71
for (int i=0;i<4;i++)
{
72
//得到随机产生的验证码数字。
73
String strRand=String.valueOf(random.nextInt(10));
74
75
//产生随机的颜色分量来构造颜色值,这样输出的每位数字的颜色值都将不同。
76
red=random.nextInt(110);
77
green=random.nextInt(50);
78
blue=random.nextInt(50);
79
80
//用随机产生的颜色将验证码绘制到图像中。
81
g.setColor(new Color(red,green,blue));
82
g.drawString(strRand,13*i+6,16);
83
84
//将产生的四个随机数组合在一起。
85
randomCode.append(strRand);
86
}
87
//将四位数字的验证码保存到Session中。
88
HttpSession session=req.getSession();
89
session.setAttribute("randomCode",randomCode.toString());
90
91
//禁止图像缓存。
92
resp.setHeader("Pragma","no-cache");
93
resp.setHeader("Cache-Control","no-cache");
94
resp.setDateHeader("Expires", 0);
95
96
resp.setContentType("image/jpeg");
97
98
//将图像输出到Servlet输出流中。
99
ServletOutputStream sos=resp.getOutputStream();
100
ImageIO.write(buffImg, "jpeg",sos);
101
sos.close();
102
}
103
}
104
105
1
写好后在web.xml中添加对应的servlet。然后页面调用的时候用。
2
<%
@page contentType="text/html"%>
3
<%
@page pageEncoding="UTF-8"%>
4
<%
--
5
The taglib directive below imports the JSTL library. If you uncomment it,
6
you must also add the JSTL library to the project. The Add Library
action
7
on Libraries node in Projects view can be used to add the JSTL 1.1 library.
8
--%>
9
<%
--
10
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
11
--%>
12
<%
13
if(request.getParameter("random")!=null)
14
{
15
if(request.getParameter("random").equals(session.getAttribute("randomCode")))
16
{
17
out.println("ok!");
18
}
19
}
20
%>
21
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
22
"http://www.w3.org/TR/html4/loose.dtd">
23
24
<html>
25
<head><title>登录页面</title>
26
<body>
27
<form method="POST" action="index.jsp">
28
<table>
29
<tr>
30
<td>用户名:</td>
31
<td><input type="text" name="username"></td>
32
</tr>
33
<tr>
34
<td>密码:</td>
35
<td><input type="password" name="password"></td>
36
</tr>
37
<tr>
38
<td>验证码:</td>
39
<td>
40
<input type="text" name="random" maxlength="4">
41
<img src="imgcode">
42
</td>
43
</tr>
44
<tr>
45
<td><input type="reset" value="重填"></td>
46
<td><input type="submit" value="提交"></td>
47
</tr>
48
</table>
49
</form>
50
</body>
51
</html>
52
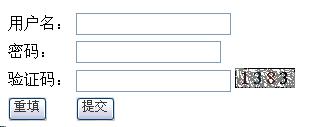
最近用netbeans 总容易发生一个运行错误,指向
<nbdeploy debugmode="false" clientUrlPart="${client.urlPart}" forceRedeploy="${forceRedeploy}"/>
。感觉是绑定tomcat失败。今天晚上调式这个程序的时候又遇到这个问题。发现重启电脑后,就正常了。也许是先前运行了其他tomcat下的程序吧。
到sun的网站看看
http://forum.java.sun.com/thread.jspa?threadID=610420&messageID=4240787
发现不少人遇到同样问题,但是具体似乎没有说出个所以然,我也不想深究,必定问题自己能解决就行。呵呵。看来netbeans M9还是不够好哦。期待beta版本。
感觉M9 运行,或者编译都显得很慢。呵呵,也许是我电脑内存只有 512M 的原因吧。
posted on 2007-05-29 02:13
-274°C 阅读(4445)
评论(3) 编辑 收藏 所属分类:
JSP 、
计算机综合