此类是一个swing选择日期控件。
效果如下图:


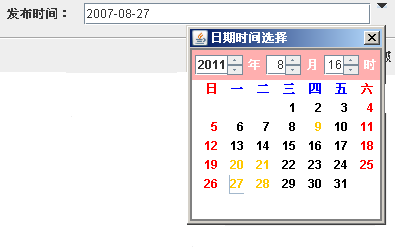
1
//
日期按钮类
2
@SuppressWarnings(
"
serial
"
)
3
public
class
DateChooserJButton
extends
JButton
{
4
5
private
DateChooser dateChooser;
6
7
private
JTextComponent com;
8
9
private
String preLabel
=
""
;
10
11
public
void
setJTextComponent(JTextComponent com)
{
12
this
.com
=
com;
13
}
14
15
public
DateChooserJButton()
{
16
this
(
null
, getNowDate(),
null
);
17
}
18
19
public
DateChooserJButton(String text)
{
20
this
(text, getNowDate(),
null
);
21
}
22
23
public
DateChooserJButton(Icon icon)
{
24
this
(
null
, getNowDate(), icon);
25
}
26
27
public
DateChooserJButton(Date date)
{
28
this
(
null
, date,
null
);
29
}
30
31
public
DateChooserJButton(String text, Date date, Icon icon)
{
32
if
(text
!=
null
)
33
super
.setText(text);
34
if
(icon
!=
null
)
35
super
.setIcon(icon);
36
setBorder(
null
);
37
setCursor(
new
Cursor(Cursor.HAND_CURSOR));
38
super
.addActionListener(
new
ActionListener()
{
39
public
void
actionPerformed(ActionEvent e)
{
40
if
(dateChooser
==
null
)
41
dateChooser
=
new
DateChooser();
42
Point p
=
getLocationOnScreen();
43
p.y
=
p.y
+
30
;
44
dateChooser.showDateChooser(p);
45
}
46
}
);
47
}
48
49
public
DateChooserJButton(SimpleDateFormat df, String dateString)
{
50
this
();
51
setText(df, dateString);
52
}
53
54
private
static
Date getNowDate()
{
55
return
Calendar.getInstance().getTime();
56
}
57
58
private
static
SimpleDateFormat getDefaultDateFormat()
{
59
return
new
SimpleDateFormat(
"
yyyy-MM-dd
"
);
60
}
61
62
public
void
setText(SimpleDateFormat df, String s)
{
63
Date date;
64
try
{
65
date
=
df.parse(s);
66
}
catch
(ParseException e)
{
67
date
=
getNowDate();
68
}
69
setDate(date);
70
}
71
72
public
void
setDate(Date date)
{
73
com.setText(getDefaultDateFormat().format(date));
74
}
75
76
public
Date getDate()
{
77
String dateString
=
getText().substring(preLabel.length());
78
try
{
79
return
getDefaultDateFormat().parse(dateString);
80
}
catch
(ParseException e)
{
81
return
getNowDate();
82
}
83
}
84
85
//
覆盖父类的方法使之无效
86
public
void
addActionListener(ActionListener listener)
{
87
}
88
89
private
class
DateChooser
extends
JPanel
implements
ActionListener,
90
ChangeListener
{
91
int
startYear
=
1980
;
//
默认【最小】显示年份
92
93
int
lastYear
=
2050
;
//
默认【最大】显示年份
94
95
int
width
=
200
;
//
界面宽度
96
97
int
height
=
200
;
//
界面高度
98
99
Color backGroundColor
=
Color.gray;
//
底色
100
101
//
月历表格配色----------------
//
102
103
Color palletTableColor
=
Color.white;
//
日历表底色
104
105
Color todayBackColor
=
Color.orange;
//
今天背景色
106
107
Color weekFontColor
=
Color.blue;
//
星期文字色
108
109
Color dateFontColor
=
Color.black;
//
日期文字色
110
111
Color weekendFontColor
=
Color.red;
//
周末文字色
112
113
//
控制条配色------------------
//
114
Color controlLineColor
=
Color.pink;
//
控制条底色
115
116
Color controlTextColor
=
Color.white;
//
控制条标签文字色
117
118
Color rbFontColor
=
Color.white;
//
RoundBox文字色
119
120
Color rbBorderColor
=
Color.red;
//
RoundBox边框色
121
122
Color rbButtonColor
=
Color.pink;
//
RoundBox按钮色
123
124
Color rbBtFontColor
=
Color.red;
//
RoundBox按钮文字色
125
126
JDialog dialog;
127
128
JSpinner yearSpin;
129
130
JSpinner monthSpin;
131
132
JSpinner hourSpin;
133
134
JButton[][] daysButton
=
new
JButton[
6
][
7
];
135
136
DateChooser()
{
137
138
setLayout(
new
BorderLayout());
139
setBorder(
new
LineBorder(backGroundColor,
2
));
140
setBackground(backGroundColor);
141
142
JPanel topYearAndMonth
=
createYearAndMonthPanal();
143
add(topYearAndMonth, BorderLayout.NORTH);
144
JPanel centerWeekAndDay
=
createWeekAndDayPanal();
145
add(centerWeekAndDay, BorderLayout.CENTER);
146
147
}
148
149
private
JPanel createYearAndMonthPanal()
{
150
Calendar c
=
getCalendar();
151
int
currentYear
=
c.get(Calendar.YEAR);
152
int
currentMonth
=
c.get(Calendar.MONTH)
+
1
;
153
int
currentHour
=
c.get(Calendar.HOUR_OF_DAY);
154
155
JPanel result
=
new
JPanel();
156
result.setLayout(
new
FlowLayout());
157
result.setBackground(controlLineColor);
158
159
yearSpin
=
new
JSpinner(
new
SpinnerNumberModel(currentYear,
160
startYear, lastYear,
1
));
161
yearSpin.setPreferredSize(
new
Dimension(
48
,
20
));
162
yearSpin.setName(
"
Year
"
);
163
yearSpin.setEditor(
new
JSpinner.NumberEditor(yearSpin,
"
####
"
));
164
yearSpin.addChangeListener(
this
);
165
result.add(yearSpin);
166
167
JLabel yearLabel
=
new
JLabel(
"
年
"
);
168
yearLabel.setForeground(controlTextColor);
169
result.add(yearLabel);
170
171
monthSpin
=
new
JSpinner(
new
SpinnerNumberModel(currentMonth,
1
,
172
12
,
1
));
173
monthSpin.setPreferredSize(
new
Dimension(
35
,
20
));
174
monthSpin.setName(
"
Month
"
);
175
monthSpin.addChangeListener(
this
);
176
result.add(monthSpin);
177
178
JLabel monthLabel
=
new
JLabel(
"
月
"
);
179
monthLabel.setForeground(controlTextColor);
180
result.add(monthLabel);
181
182
hourSpin
=
new
JSpinner(
new
SpinnerNumberModel(currentHour,
0
,
23
,
183
1
));
184
hourSpin.setPreferredSize(
new
Dimension(
35
,
20
));
185
hourSpin.setName(
"
Hour
"
);
186
hourSpin.addChangeListener(
this
);
187
result.add(hourSpin);
188
189
JLabel hourLabel
=
new
JLabel(
"
时
"
);
190
hourLabel.setForeground(controlTextColor);
191
result.add(hourLabel);
192
193
return
result;
194
}
195
196
private
JPanel createWeekAndDayPanal()
{
197
String colname[]
=
{
"
日
"
,
"
一
"
,
"
二
"
,
"
三
"
,
"
四
"
,
"
五
"
,
"
六
"
}
;
198
JPanel result
=
new
JPanel();
199
//
设置固定字体,以免调用环境改变影响界面美观
200
result.setFont(
new
Font(
"
宋体
"
, Font.PLAIN,
12
));
201
result.setLayout(
new
GridLayout(
7
,
7
));
202
result.setBackground(Color.white);
203
JLabel cell;
204
205
for
(
int
i
=
0
; i
<
7
; i
++
)
{
206
cell
=
new
JLabel(colname[i]);
207
cell.setHorizontalAlignment(JLabel.RIGHT);
208
if
(i
==
0
||
i
==
6
)
209
cell.setForeground(weekendFontColor);
210
else
211
cell.setForeground(weekFontColor);
212
result.add(cell);
213
}
214
215
int
actionCommandId
=
0
;
216
for
(
int
i
=
0
; i
<
6
; i
++
)
217
for
(
int
j
=
0
; j
<
7
; j
++
)
{
218
JButton numberButton
=
new
JButton();
219
numberButton.setBorder(
null
);
220
numberButton.setHorizontalAlignment(SwingConstants.RIGHT);
221
numberButton.setActionCommand(String
222
.valueOf(actionCommandId));
223
numberButton.addActionListener(
this
);
224
numberButton.setBackground(palletTableColor);
225
numberButton.setForeground(dateFontColor);
226
if
(j
==
0
||
j
==
6
)
227
numberButton.setForeground(weekendFontColor);
228
else
229
numberButton.setForeground(dateFontColor);
230
daysButton[i][j]
=
numberButton;
231
result.add(numberButton);
232
actionCommandId
++
;
233
}
234
235
return
result;
236
}
237
238
private
JDialog createDialog(Frame owner)
{
239
JDialog result
=
new
JDialog(owner,
"
日期时间选择
"
,
true
);
240
result.setDefaultCloseOperation(JDialog.HIDE_ON_CLOSE);
241
result.getContentPane().add(
this
, BorderLayout.CENTER);
242
result.pack();
243
result.setSize(width, height);
244
return
result;
245
}
246
247
@SuppressWarnings(
"
deprecation
"
)
248
void
showDateChooser(Point position)
{
249
Frame owner
=
(Frame) SwingUtilities
250
.getWindowAncestor(DateChooserJButton.
this
);
251
if
(dialog
==
null
||
dialog.getOwner()
!=
owner)
252
dialog
=
createDialog(owner);
253
dialog.setLocation(getAppropriateLocation(owner, position));
254
flushWeekAndDay();
255
dialog.show();
256
}
257
258
Point getAppropriateLocation(Frame owner, Point position)
{
259
Point result
=
new
Point(position);
260
Point p
=
owner.getLocation();
261
int
offsetX
=
(position.x
+
width)
-
(p.x%
posted on 2007-08-15 14:30
月月鸟 阅读(6630)
评论(13) 编辑 收藏 所属分类:
J2SE