Posted on 2009-02-27 15:48
啥都写点 阅读(1167)
评论(0) 编辑 收藏 所属分类:
J2EE
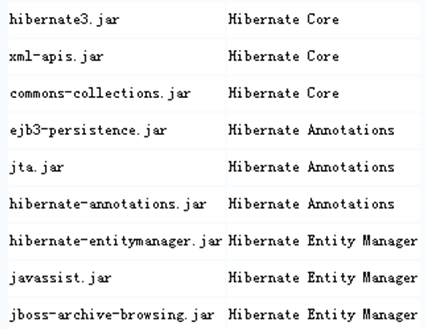
Person类代码
package quickstart.model;import javax.persistence.Entity;import javax.persistence.GeneratedValue;import javax.persistence.Id;
@Entity
public class Person {
@Id
@GeneratedValue
private Integer id;
private String lastName;
private String firstName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
} public String getLastName() {
return lastName;
} public void setLastName(String lastName) {
this.lastName = lastName;
} public Integer getId() {
return id;
} public void setId(Integer id) {
this.id = id;
}
}
创建一个接口,命名为"PersonService",包名为"quickstart.service" PersonService.java
package quickstart.service;import java.util.List;import quickstart.model.Person;public interface PersonService {
public List<Person> findAll();
public void save(Person person);
public void remove(int id);
public Person find(int id);}
创建一个类,命名为"PersonServiceImpl",包名为"quickstart.service"PersonServiceImpl.java
package quickstart.service;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import org.springframework.transaction.annotation.Transactional;
import quickstart.model.Person;
@Transactional
public class PersonServiceImpl implements PersonService {
private EntityManager em;
@PersistenceContext
public void setEntityManager(EntityManager em) {
this.em = em;
}
@SuppressWarnings("unchecked")
public List<Person> findAll() {
Query query = getEntityManager().createQuery("select p FROM Person p");
return query.getResultList();
}
public void save(Person person) {
if (person.getId() == null) {
// new em.persist(person);
} else {
// update em.merge(person); }
}
public void remove(int id) {
Person person = find(id);
if (person != null) {
em.remove(person);
}
}
private EntityManager getEntityManager() {
return em;
}
public Person find(int id) {
return em.find(Person.class, id);
}
}
@PersistenceContext会让Spring在实例化的时候给服务注入一个EntityManager。@PersistenceContext注解可以放在实例变量,或者setter方法前面。如果一个类被注解为@Transactional,Spring将会确保类的方法在运行在一个事务中。
JPA配置
persistence.xml中就不用配置了,因为会教给spring保管
web.xml的配置
<?xml version="1.0" encoding="UTF-8"?>
<web-app id="person" version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <display-name>person</display-name>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.FilterDispatcher</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app>
applicationContext.xml配置如下:
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.0.xsd">
<bean class="org.springframework.orm.jpa.support.PersistenceAnnotationBeanPostProcessor" />
<bean id="personService" class="quickstart.service.PersonServiceImpl" />
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="jpaVendorAdapter">
<bean class="org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter">
<property name="database" value="MYSQL" />
<property name="showSql" value="true" />
</bean>
</property>
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost/test" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<bean id="transactionManager" class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory" ref="entityManagerFactory" />
</bean>
<tx:annotation-driven transaction-manager="transactionManager" />
<bean id="personAction" scope="prototype" class="quickstart.action.PersonAction">
<constructor-arg ref="personService" />
</bean>
</beans>
注意"personAction"bean的"class"属性被设为Action类的名字,并且"personService"bean会作为参数传递到action的构造器中。改变"dataSource"Bean的"url",
"username"和"passwrod"属性为你数据库的值。更多beans设置的细节,请参看Spring的文档。"scope"是Spring2新增的属性,它意味着Spring会在该类型的对象被请求时创建一个新的PersonAction对象。在Struts2里,一个新的action对象被创建,用来为每个请求服务,这就是我们为什么需要scope="prototype"。
现在我们需要创建一个简单的Struts action,它封装了PersonServices的方法。并且我们配置Struts使用Spring作为对象工厂。
打开新建类对话框,输入"PersonAction"作为类名,包名为"quickstart.action",内容如下:
package quickstart.action;
import java.util.List;
import quickstart.model.Person;
import quickstart.service.PersonService;
import com.opensymphony.xwork2.Action;
import com.opensymphony.xwork2.Preparable;
public class PersonAction implements Preparable {
private PersonService service;
private List<Person> persons;
private Person person;
private Integer id;
public PersonAction(PersonService service) {
this.service = service;
}
public String execute() {
this.persons = service.findAll();
return Action.SUCCESS;
}
public String save() {
this.service.save(person);
this.person = new Person();
return execute();
}
public String remove() {
service.remove(id);
return execute();
}
public List<Person> getPersons() {
return persons;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public void prepare() throws Exception {
if (id != null)
person = service.find(id);
}
public Person getPerson() {
return person;
}
public void setPerson(Person person) {
this.person = person;
}
}
struts.xml中的配置
<?xml version="1.0" encoding="UTF-8" ?><!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"><struts>
<constant name="struts.objectFactory" value="spring" />
<constant name="struts.devMode" value="true" />
<package name="person" extends="struts-default">
<action name="list" method="execute" class="personAction">
<result>pages/list.jsp</result>
<result name="input">pages/list.jsp</result>
</action>
<action name="remove" class="personAction" method="remove">
<result>pages/list.jsp</result>
<result name="input">pages/list.jsp</result>
</action>
<action name="save" class="personAction" method="save">
<result>pages/list.jsp</result>
<result name="input">pages/list.jsp</result>
</action>
</package>
</struts>
设置"struts.objectFactory"为"spring"会强制Struts使用Spring来实例化action,并注入所有定义在applicationContext.xml中的依赖关系。每个action别名的"class"属性被设置为"personAction",这也就是我们在applicationContext.xml中定义的PersonAction bean。要让Struts与Spring一起工作,我们仅仅需要做上面这点事情。
--
学海无涯