第三篇:http://blog.csdn.net/mapdigit/article/details/7555429App->Activity->Animation这个Demo主要讲的是两个Activity在跳转过程中的动画应用。
通过Android Manifest.xml文件找到com.example.android.apis.app包下的 Animation类:
1
<activity android:name=".app.Animation" android:label="@string/activity_animation">
2
<intent-filter>
3
<action android:name="android.intent.action.MAIN" />
4
<category android:name="android.intent.category.SAMPLE_CODE" />
5
</intent-filter>
6
</activity> Animation.java类:
在onCreate()方法中:
1
@Override
2
protected void onCreate(Bundle savedInstanceState)
{
3
super.onCreate(savedInstanceState);
4
5
setContentView(R.layout.activity_animation);
6
7
// Watch for button clicks.
8
Button button = (Button)findViewById(R.id.fade_animation);
9
button.setOnClickListener(mFadeListener);
10
button = (Button)findViewById(R.id.zoom_animation);
11
button.setOnClickListener(mZoomListener);
12
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.JELLY_BEAN)
{
13
button = (Button)findViewById(R.id.modern_fade_animation);
14
button.setOnClickListener(mModernFadeListener);
15
button = (Button)findViewById(R.id.modern_zoom_animation);
16
button.setOnClickListener(mModernZoomListener);
17
button = (Button)findViewById(R.id.scale_up_animation);
18
button.setOnClickListener(mScaleUpListener);
19
button = (Button)findViewById(R.id.zoom_thumbnail_animation);
20
button.setOnClickListener(mZoomThumbnailListener);
21
} else
{
22
findViewById(R.id.modern_fade_animation).setEnabled(false);
23
findViewById(R.id.modern_zoom_animation).setEnabled(false);
24
findViewById(R.id.scale_up_animation).setEnabled(false);
25
findViewById(R.id.zoom_thumbnail_animation).setEnabled(false);
26
}
27
}
android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.JELLY_BEAN
判断当前版本是否大于等于16 ,Build类可以获取很多手机信息。
判断是因为下面的四个Button是JellyBean的新特性,如果是其他的版本那么这四个按钮的不可用的。
找到activity_animation.xml,该界面有一个TextView,六个Button
1
<?xml version="1.0" encoding="utf-8"?>
2
<!-- Copyright (C) 2009 The Android Open Source Project
3
4
Licensed under the Apache License, Version 2.0 (the "License");
5
you may not use this file except in compliance with the License.
6
You may obtain a copy of the License at
7
8
http://www.apache.org/licenses/LICENSE-2.0
9
10
Unless required by applicable law or agreed to in writing, software
11
distributed under the License is distributed on an "AS IS" BASIS,
12
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
13
See the License for the specific language governing permissions and
14
limitations under the License.
15
-->
16
17
<!-- Demonstrates starting and stopping a local service.
18
See corresponding Java code com.android.sdk.app.LocalSerice.java. -->
19
20
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:padding="4dip"
21
android:gravity="center_horizontal"
22
android:layout_width="match_parent" android:layout_height="match_parent">
23
24
<TextView
25
android:layout_width="match_parent" android:layout_height="wrap_content"
26
android:layout_weight="0" android:paddingBottom="4dip"
27
android:textAppearance="?android:attr/textAppearanceMedium"
28
android:text="@string/activity_animation_msg"/>
29
30
<Button android:id="@+id/fade_animation"
31
android:layout_width="wrap_content" android:layout_height="wrap_content"
32
android:text="@string/activity_animation_fade">
33
<requestFocus />
34
</Button>
35
36
<Button android:id="@+id/zoom_animation"
37
android:layout_width="wrap_content" android:layout_height="wrap_content"
38
android:text="@string/activity_animation_zoom">
39
</Button>
40
41
<Button android:id="@+id/modern_fade_animation"
42
android:layout_width="wrap_content" android:layout_height="wrap_content"
43
android:text="@string/activity_modern_animation_fade">
44
<requestFocus />
45
</Button>
46
47
<Button android:id="@+id/modern_zoom_animation"
48
android:layout_width="wrap_content" android:layout_height="wrap_content"
49
android:text="@string/activity_modern_animation_zoom">
50
</Button>
51
52
<Button android:id="@+id/scale_up_animation"
53
android:layout_width="wrap_content" android:layout_height="wrap_content"
54
android:text="@string/activity_scale_up_animation">
55
</Button>
56
57
<Button android:id="@+id/zoom_thumbnail_animation"
58
android:layout_width="wrap_content" android:layout_height="wrap_content"
59
android:text="@string/activity_zoom_thumbnail_animation">
60
</Button>
61
62
</LinearLayout>
63
64
我们发现在有的Button上有<requestFocus />标签
该标签用于指定屏幕内的焦点View。
例如我们点击tab键或enter键焦点自动进入下一个输入框
用法: 将标签置于Views标签内部
1
<EditText id="@+id/text"
2
android:layout_width="fill_parent"
3
android:layout_height="wrap_content"
4
android:layout_weight="0"
5
android:paddingBottom="4">
6
<requestFocus />
7
</EditText>
ml布局里面设置文字的外观:
如“android:textAppearance=“?android:attr/textAppearanceLargeInverse”这里引用的是系统自带的一个外观,
?表示系统是否有这种外观,否则使用默认的外观。
现在再让我们回到Animation类,
Android 中 Animation 资源可以分为两种:
- Tween Animation 对单个图像进行各种变换(缩放,平移,旋转等)来实现动画。
- Frame Animation 由一组图像顺序显示显示动画。
Animation 中使用的是Tween Animation,使用的资源为R.anim.fade, R.anim.hold,R.anim.zoom_enter, R.anim.zoom_exit:
第一按钮:
fade.xml:
1
<?xml version="1.0" encoding="utf-8"?>
2
<!-- Copyright (C) 2007 The Android Open Source Project
3
4
Licensed under the Apache License, Version 2.0 (the "License");
5
you may not use this file except in compliance with the License.
6
You may obtain a copy of the License at
7
8
http://www.apache.org/licenses/LICENSE-2.0
9
10
Unless required by applicable law or agreed to in writing, software
11
distributed under the License is distributed on an "AS IS" BASIS,
12
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
13
See the License for the specific language governing permissions and
14
limitations under the License.
15
-->
16
17
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
18
android:interpolator="@android:anim/accelerate_interpolator"
19
android:fromAlpha="0.0" android:toAlpha="1.0"
20
android:duration="@android:integer/config_longAnimTime" />
21
hold.xml:
1
<?xml version="1.0" encoding="utf-8"?>
2
<!-- Copyright (C) 2009 The Android Open Source Project
3
4
Licensed under the Apache License, Version 2.0 (the "License");
5
you may not use this file except in compliance with the License.
6
You may obtain a copy of the License at
7
8
http://www.apache.org/licenses/LICENSE-2.0
9
10
Unless required by applicable law or agreed to in writing, software
11
distributed under the License is distributed on an "AS IS" BASIS,
12
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
13
See the License for the specific language governing permissions and
14
limitations under the License.
15
-->
16
17
<translate xmlns:android="http://schemas.android.com/apk/res/android"
18
android:interpolator="@android:anim/accelerate_interpolator"
19
android:fromXDelta="0" android:toXDelta="0"
20
android:duration="@android:integer/config_longAnimTime" />
21
zoom_enter.xml:
1
<?xml version="1.0" encoding="utf-8"?>
2
<!--
3
/*
4
** Copyright 2009, The Android Open Source Project
5
**
6
** Licensed under the Apache License, Version 2.0 (the "License");
7
** you may not use this file except in compliance with the License.
8
** You may obtain a copy of the License at
9
**
10
** http://www.apache.org/licenses/LICENSE-2.0
11
**
12
** Unless required by applicable law or agreed to in writing, software
13
** distributed under the License is distributed on an "AS IS" BASIS,
14
** WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
15
** See the License for the specific language governing permissions and
16
** limitations under the License.
17
*/
18
-->
19
20
<!-- Special window zoom animation: this is the element that enters the screen,
21
it starts at 200% and scales down. Goes with zoom_exit.xml. -->
22
<set xmlns:android="http://schemas.android.com/apk/res/android"
23
android:interpolator="@android:anim/decelerate_interpolator">
24
<scale android:fromXScale="2.0" android:toXScale="1.0"
25
android:fromYScale="2.0" android:toYScale="1.0"
26
android:pivotX="50%p" android:pivotY="50%p"
27
android:duration="@android:integer/config_mediumAnimTime" />
28
</set>
29
zoom_exit.xml:
1
<?xml version="1.0" encoding="utf-8"?>
2
<!--
3
/*
4
** Copyright 2009, The Android Open Source Project
5
**
6
** Licensed under the Apache License, Version 2.0 (the "License");
7
** you may not use this file except in compliance with the License.
8
** You may obtain a copy of the License at
9
**
10
** http://www.apache.org/licenses/LICENSE-2.0
11
**
12
** Unless required by applicable law or agreed to in writing, software
13
** distributed under the License is distributed on an "AS IS" BASIS,
14
** WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
15
** See the License for the specific language governing permissions and
16
** limitations under the License.
17
*/
18
-->
19
20
<!-- Special window zoom animation: this is the element that exits the
21
screen, it is forced above the entering element and starts at its
22
normal size (filling the screen) and scales down while fading out.
23
This goes with zoom_enter.xml. -->
24
<set xmlns:android="http://schemas.android.com/apk/res/android"
25
android:interpolator="@android:anim/decelerate_interpolator"
26
android:zAdjustment="top">
27
<scale android:fromXScale="1.0" android:toXScale=".5"
28
android:fromYScale="1.0" android:toYScale=".5"
29
android:pivotX="50%p" android:pivotY="50%p"
30
android:duration="@android:integer/config_mediumAnimTime" />
31
<alpha android:fromAlpha="1.0" android:toAlpha="0"
32
android:duration="@android:integer/config_mediumAnimTime"/>
33
</set>
34
从代码可以看到Activity Animation到其它Activity Controls1 切换的动画使用overridePendingTransition 来定义,函数overridePendingTransition(int enterAnim, int exitAnim) 必须定义在StartActivity(Intent)或是 Activity.finish()之后来定义两个Activity切换时的动画,enterAnim 为新Activity出现时动画效果,exitAnim则定义了当前Activity退出时动画效果。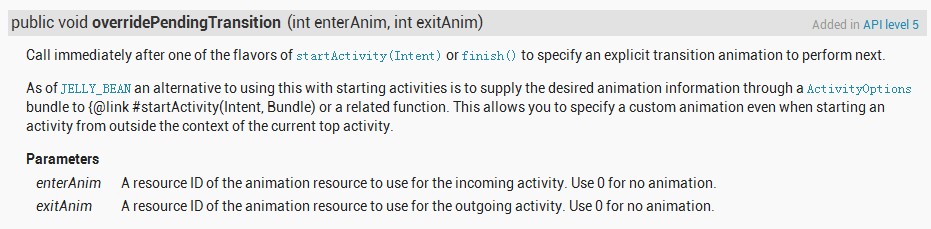
第三个按钮和第四个按钮的动画分别与第一个按钮和第二个动画相同,只不过换了一种实现的方式。利用API16的ActivityOptions类。
通过ActivityOptions设置动画转化为Bundle,作为Intent属性启动activity。由一个activity的启动、另一个activity的停止两组动画组成。
http://developer.android.com/reference/android/app/ActivityOptions.html
下面三个按钮都是通过ActivityOptions的三个方法来创建动画。
最后一个动画是通过在指定某个地方放入一个新Activity的缩略图,然后充满充满整个屏幕。

View组件显示的内容可以通过cache机制保存为bitmap, 使用到的api有
void setDrawingCacheEnabled(boolean flag),
Bitmap getDrawingCache(boolean autoScale),
void buildDrawingCache(boolean autoScale),
void destroyDrawingCache()
我们要获取它的cache先要通过setDrawingCacheEnable方法把cache开启,然后再调用getDrawingCache方法就可以获得view的cache图片了。buildDrawingCache方法可以不用调用,因为调用getDrawingCache方法时,若果cache没有建立,系统会自动调用buildDrawingCache方法生成cache。若果要更新cache, 必须要调用destoryDrawingCache方法把旧的cache销毁,才能建立新的。
当调用setDrawingCacheEnabled方法设置为false, 系统也会自动把原来的cache销毁。
ViewGroup在绘制子view时,而外提供了两个方法
void setChildrenDrawingCacheEnabled(boolean enabled)
setChildrenDrawnWithCacheEnabled(boolean enabled)
setChildrenDrawingCacheEnabled方法可以使viewgroup里所有的子view开启cache, setChildrenDrawnWithCacheEnabled使在绘制子view时,若该子view开启了cache, 则使用它的cache进行绘制,从而节省绘制时间。
获取cache通常会占用一定的内存,所以通常不需要的时候有必要对其进行清理,通过destroyDrawingCache或setDrawingCacheEnabled(false)实现。