复习题
1、哪一存储类生成的变量对于包含他们的函数来说是局部变量?
答:自动存储类、寄存器存储类和静态空链接存储类
2、哪一存储类的变量在包含它们的程序运行时期内一直存在?
答:静态空链接存储类、静态内部链接存储类和静态外部链接存储类
3、哪一存储类的变量可以在多个文件中使用?哪一存储类的变量只限于在一个文件中使用?
答:静态外部链接存储类和静态内部链接存储类
4、代码块作用域变量具有哪种链接?
答:空链接
5、关键字extern的用处是什么?
答:在声明中使用关键字extern表明一个变量或函数已经在其他地方被定义过了
6、考虑如下代码段:
int * p1 = (int *)malloc(100 * sizeof(int));
考虑到最终的结果,下面的语句有何不同?
int * p1 = (int *)calloc(100, sizeof(int));
答:都分配一个具有100个int值的数组。使用calloc()的语句还把每个元素设置为0
7、下列每个变量对哪些函数是可见的?程序有什么错误吗?
/* 文件1 */int daisy;
int main(
void)
{
int lily;

;
}
int petal()
{
extern int daisy, lily;

;
}
/* 文件2 */extern int daisy;
static int lily;
int rose;
int stem()
{
int rose;

;
}
void root()
{

;
}
答:
daisy对main()是默认可见的,对petal()、stem()和root()是通过extern声明可见的。文件2中的声明extern int daisy;使得daisy对该文件中的所有函数可见。第一个lily是main()的局部变量。petal()中对lily的引用是错误的,因为两个文件中都没有lily的外部声明。有一个外部的静态lily,但是它只对第二个文件中的函数可见。第一个外部rose对root()可见,但是stem()使用它自己的局部rose覆盖了外部的rose。
8、下面程序会打印出什么?
#include <stdio.h>
char color = 'B';
void first(void);
void second(void);
int main(void)
{
extern char color;
printf("color in main() is %c\n", color);
first();
printf("color in main() is %c\n", color);
second();
printf("color in main() is %c\n", color);
return 0;
}
void first(void)
{
char color;
color = 'R';
printf("color in first() is %c\n", color);
}
void second(void)
{
color = 'G';
printf("color in second() is %c\n", color);
}
答:
color in main() is B
color in first() is R
color in main() is B
color in second() is G
color in main() is G
9、文件开始处做了如下声明:
static int plink;
int value_ct(const int arr[], int value, int n);
a.这些声明表明了程序员的什么意图?
b.用const int value和const int n代替int value和int n会增强对调用程序中的值的保护吗?
答:
a.它告诉我们程序将使用一个变量plink,该变量局部于包含该函数的文件。value_ct()的第一个参数是一个指向整数的指针,并假定它指向具有n个元素的数组的第一个元素。这里重要的一点是不允许程序使用指针arr来修改原始数组的值。
b.不会。value和n已经是原始数据的拷贝,所以函数不能改变调用程序中的对应值。这样声明起到的作用只是防止在函数中改变value和n的值。例如,如果const限定n,那么函数就不能使用n++表达式。
编程练习1、
#include <stdio.h>
int critic(void);
int main(void)
{
int units;
printf("How many pounds to a firkin of butter?\n");
scanf("%d", &units);
while(units != 56)
units = critic();
printf("You must have looked it up!\n");
return 0;
}
int critic(void)
{
int units;
printf("No luck, chummy. Try again.\n");
scanf("%d", &units);
return units;
}
2、
/* pe12-2b.c */
#include <stdio.h>
#include "pe12-2a.h"
int main(void)
{
int mode;
printf("Enter 0 for metric mode, 1 for US mode: ");
scanf("%d", &mode);
while(mode >= 0)
{
set_mode(mode);
get_info();
show_info();
printf("Enter 0 for metric mode, 1 for US mode");
printf(" (-1 to quit): ");
scanf("%d", &mode);
}
printf("Done.\n");
return 0;
}
/* pe12-2a.c */
#include <stdio.h>
#include <stdlib.h>
static int mode; // 代表模式
static int distance; // 代表距离
static double fuel_consumption; // 代表消耗的燃料
// 设置模式
void set_mode(int m)
{
mode = m;
}
// 根据模式设置提示输入相应的数据
void get_info(void)
{
if(mode == 0) // 公制
{
printf("Enter distance traveled in kilometers: ");
scanf("%d", &distance);
printf("Enter fuel consumed in liters: ");
scanf("%lf", &fuel_consumption);
}
else if(mode == 1) // 美制
{
printf("Enter distance traveled in miles: ");
scanf("%d", &distance);
printf("Enter fuel consumed in gallons: ");
scanf("%lf", &fuel_consumption);
}
else
{
printf("Invalid mode specified. Mode 1 (US) used.\n");
printf("Enter distance traveled in miles: ");
scanf("%d", &distance);
printf("Enter fuel consumed in gallons: ");
scanf("%lf", &fuel_consumption);
}
}
// 根据所选的模式计算并显示燃料消耗值
void show_info(void)
{
if(mode == 0)
printf("Fuel consumption is %.2f liters per 100 km.\n", fuel_consumption / (distance / 100));
else
printf("Fuel consumption is %.1f miles per gallon.\n", distance / fuel_consumption);
}
/* pe12-2a.h */
void set_mode(int mode);
// 根据模式设置提示输入相应的数据
void get_info(void);
// 根据所选的模式计算并显示燃料消耗值
void show_info(void);
3、
/* pe12-2b.c */
#include <stdio.h>
#include "pe12-2a.h"
int main(void)
{
int mode;
printf("Enter 0 for metric mode, 1 for US mode: ");
scanf("%d", &mode);
while(mode >= 0)
{
//set_mode(mode);
get_info(mode);
//show_info(mode);
printf("Enter 0 for metric mode, 1 for US mode");
printf(" (-1 to quit): ");
scanf("%d", &mode);
}
printf("Done.\n");
return 0;
}
/* pe12-2a.c */
#include <stdio.h>
#include <stdlib.h>
// 根据模式设置提示输入相应的数据
void get_info(int mode)
{
int distance; // 代表距离
double fuel_consumption; // 代表消耗的燃料
double result; // 计算油耗
if(mode == 0) // 公制
{
printf("Enter distance traveled in kilometers: ");
scanf("%d", &distance);
printf("Enter fuel consumed in liters: ");
scanf("%lf", &fuel_consumption);
result = fuel_consumption / (distance / 100);
printf("Fuel consumption is %.2f liters per 100 km.\n", result);
}
else if(mode == 1) // 美制
{
printf("Enter distance traveled in miles: ");
scanf("%d", &distance);
printf("Enter fuel consumed in gallons: ");
scanf("%lf", &fuel_consumption);
result = distance / fuel_consumption;
printf("Fuel consumption is %.1f miles per gallon.\n", result);
}
else
{
printf("Invalid mode specified. Mode 1 (US) used.\n");
printf("Enter distance traveled in miles: ");
scanf("%d", &distance);
printf("Enter fuel consumed in gallons: ");
scanf("%lf", &fuel_consumption);
result = distance / fuel_consumption;
printf("Fuel consumption is %.1f miles per gallon.\n", result);
}
}
/* pe12-2a.h */
// 根据模式设置提示输入相应的数据
double get_info(int mode);
4、
#include <stdio.h>
int helloworld(void);
static int count = 0;
int main(void)
{
char ch;
printf("Please enter a character (q to quit): ");
while((ch = getchar()) != 'q')
{
count = helloworld();
while(getchar() != '\n')
continue;
printf("Please enter a character (q to quit): ");
}
printf("Number of times the function helloworld() itself is called: %d\n", count);
return 0;
}
int helloworld(void)
{
count++;
return count;
}
程序应尽量写的短一点,第二次更新如下:
#include <stdio.h>
void sayhello(void);
static int count = 0;
int main(void)
{
for(int n = 0; n < 7; n++)
sayhello();
printf("函数sayhello()被调用的次数为:%d\n", count);
return 0;
}
void sayhello(void)
{
printf("Hello World!\n");
count++;
}
5、
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 产生1~10范围内的随机数
int rollem(void);
void sort(int *array);
int main(void)
{
int arr[100];
int i;
srand((unsigned) time(0)); // 随机化种子
for(i = 0; i < 100; i++)
{
arr[i] = rollem();
}
sort(arr);
printf("The result is a descending sort: \n");
for(i = 0; i < 100; i++)
{
printf("%d ", arr[i]);
// 每行打印10个数,然后换行
if(i % 10 == 9)
putchar('\n');
}
return 0;
}
// 产生1~10范围内的随机数
int rollem(void)
{
int roll;
roll = rand() % 10 + 1;
return roll;
}
void sort(int *array)
{
int i, j, temp;
for(i = 0; i < 100 - 1; i++)
{
for(j = i + 1; j < 100; j++)
{
if(array[i] < array[j])
{
temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
}
}
一个可能的运行结果:

6、
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 产生1~10范围内的随机数
int rollem(void);
int main(void)
{
int i, random_number;
int arr[10] = { arr[0] = 0 };
srand((unsigned) time(0)); // 随机化种子
for(i = 0; i < 1000; i++)
{
random_number = rollem();
switch(random_number)
{
case 1: arr[0]++;
break;
case 2: arr[1]++;
break;
case 3: arr[2]++;
break;
case 4: arr[3]++;
break;
case 5: arr[4]++;
break;
case 6: arr[5]++;
break;
case 7: arr[6]++;
break;
case 8: arr[7]++;
break;
case 9: arr[8]++;
break;
case 10: arr[9]++;
break;
}
}
for(i = 0; i < 10; i++)
printf("Numbers of random number %d is %d\n", i+1, arr[i]);
return 0;
}
// 产生1~10范围内的随机数
int rollem(void)
{
int roll;
roll = rand() % 10 + 1;
return roll;
}
一个可能的运行结果为:

7、(看来第一次就做的挺好的,没必要再次更新!!!)
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include "diceroll.h"
// 产生1~10范围内的随机数
int rollem(void);
int main(void)
{
char ch;
int dice, index = 0;
int sides, sets;
int * pti;
srand((unsigned) time(0)); // 随机化种子
printf("Enter the number of sets: enter q to stop.\n");
while(scanf("%d", &sets))
{
pti = (int *)malloc(sets * sizeof(int));
if(pti == NULL)
{
puts("Memory allocation failed. Goodbye.");
exit(EXIT_FAILURE);
}
printf("How many sides and how many dice?\n");
scanf("%d %d", &sides, &dice);
for(index = 0; index < sets; index++)
// pti现在指向有sets个元素的数组
pti[index] = roll_n_dice(dice, sides);
printf("Here are %d sets of %d %d-sided throws.\n", sets, dice, sides);
for(index = 0; index < sets; index++)
{
printf("%d ", pti[index]);
if(index % 15 == 14)
putchar('\n');
}
if(index % 15 != 0)
putchar('\n');
while((ch = getchar()) != '\n')
continue;
printf("How many sets? Enter q to stop.\n");
free(pti);
}
return 0;
}
#include "diceroll.h"
#include <stdio.h>
#include <stdlib.h>
int roll_count = 0;
static int rollem(int sides)
{
int roll;
roll = rand() % sides + 1;
++roll_count;
return roll;
}
int roll_n_dice(int dice, int sides)
{
int d;
int total = 0;
if(sides < 2)
{
printf("Need at least 2 sides.\n");
return -2;
}
if(dice < 1)
{
printf("Need at least 1 die.\n");
return -1;
}
for(d = 0; d < dice; d++)
total += rollem(sides);
return total;
}
extern int roll_count;
int roll_n_dice(int dice, int sides);
一个可能的运行结果为:

8、
// pe12-8.c
#include <stdio.h>
#include <stdlib.h>
int *make_array(int elem, int val);
void show_array(const int arr[], int n);
int main(void)
{
int *pa;
int size;
int value;
printf("Enter the number of elements: ");
scanf("%d", &size);
while(size > 0)
{
printf("Enter the initialization value: ");
scanf("%d", &value);
pa = make_array(size, value);
if(pa)
{
show_array(pa, size);
free(pa);
}
printf("Enter the number of elements (<1 to quit): ");
scanf("%d", &size);
}
printf("Done.\n");
return 0;
}
int *make_array(int elem, int val)
{
int *pti;
pti = (int *)malloc(elem * sizeof(int));
if(pti == NULL)
{
puts("Memory allocation failed. Goodbye.");
exit(EXIT_FAILURE);
}
// 为数组中的每个值赋val值
for(int i = 0; i < elem; i++)
pti[i] = val;
return pti;
}
void show_array(const int arr[], int n)
{
int i ;
for(i = 0; i < n; i++)
{
printf("%d ", arr[i]);
if(i % 8 == 7)
putchar('\n');
}
if(i % 8 != 0)
putchar('\n');
}
一个可能的运行结果为:
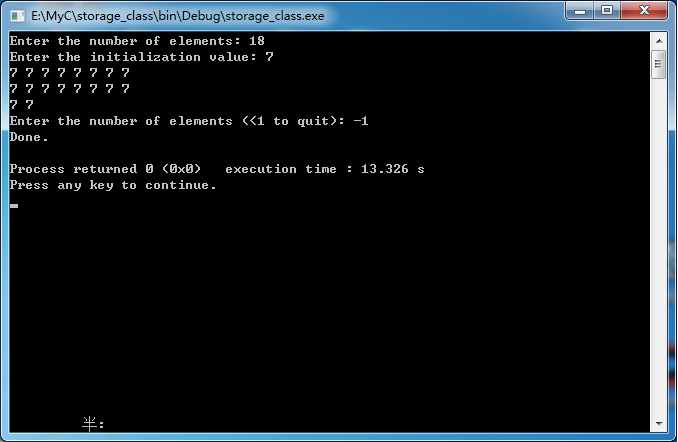
总结:这一章的编程练习还算是很简单的,自己一个人硬是磨出来的,没有借鉴任何人的做法,虽然做的慢,还算是给自己一个交代!!!