文件结构如下 开发工具myEclipse6.M1
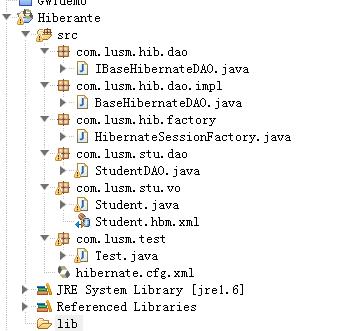
代码(具体位置看package位置)
package com.lusm.hib.dao;

import org.hibernate.Session;


public interface IBaseHibernateDAO
{
public Session getSession();
}
package com.lusm.hib.dao.impl;

import org.hibernate.Session;

import com.lusm.hib.dao.IBaseHibernateDAO;
import com.lusm.hib.factory.HibernateSessionFactory;


public class BaseHibernateDAO implements IBaseHibernateDAO
{


public Session getSession()
{
return HibernateSessionFactory.getSession();
}

}
package com.lusm.hib.factory;

import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;


public class HibernateSessionFactory
{

private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal<Session> threadLocal = new ThreadLocal<Session>();
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;


static
{

try
{
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();

} catch (Exception e)
{
System.err.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}


private HibernateSessionFactory()
{
}


public static Session getSession() throws HibernateException
{
Session session = (Session) threadLocal.get();


if (session == null || !session.isOpen())
{

if (sessionFactory == null)
{
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}

return session;
}


public static void rebuildSessionFactory()
{

try
{
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();

} catch (Exception e)
{
System.err.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}


public static void closeSession() throws HibernateException
{
Session session = (Session) threadLocal.get();
threadLocal.set(null);


if (session != null)
{
session.close();
}
}


public static org.hibernate.SessionFactory getSessionFactory()
{
return sessionFactory;
}


public static void setConfigFile(String configFile)
{
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}


public static Configuration getConfiguration()
{
return configuration;
}

}
package com.lusm.stu.dao;

import java.util.List;

import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.LockMode;
import org.hibernate.Query;
import org.hibernate.criterion.Example;

import com.lusm.hib.dao.impl.BaseHibernateDAO;
import com.lusm.stu.vo.Student;


public class StudentDAO extends BaseHibernateDAO
{
private static final Log log = LogFactory.getLog(StudentDAO.class);
// property constants
public static final String NAME = "name";


public void save(Student transientInstance)
{
log.debug("saving Student instance");

try
{
getSession().save(transientInstance);
log.debug("save successful");

} catch (RuntimeException re)
{
log.error("save failed", re);
throw re;
}
}


public void delete(Student persistentInstance)
{
log.debug("deleting Student instance");

try
{
getSession().delete(persistentInstance);
log.debug("delete successful");

} catch (RuntimeException re)
{
log.error("delete failed", re);
throw re;
}
}


public Student findById(java.lang.Long id)
{
log.debug("getting Student instance with id: " + id);

try
{
Student instance = (Student) getSession().get(
"com.lusm.stu.vo.Student", id);
return instance;

} catch (RuntimeException re)
{
log.error("get failed", re);
throw re;
}
}


public List findByExample(Student instance)
{
log.debug("finding Student instance by example");

try
{
List results = getSession().createCriteria(
"com.lusm.stu.vo.Student").add(Example.create(instance))
.list();
log.debug("find by example successful, result size: "
+ results.size());
return results;

} catch (RuntimeException re)
{
log.error("find by example failed", re);
throw re;
}
}


public List findByProperty(String propertyName, Object value)
{
log.debug("finding Student instance with property: " + propertyName
+ ", value: " + value);

try
{
String queryString = "from Student as model where model."
+ propertyName + "= ?";
Query queryObject = getSession().createQuery(queryString);
queryObject.setParameter(0, value);
return queryObject.list();

} catch (RuntimeException re)
{
log.error("find by property name failed", re);
throw re;
}
}


public List findByName(Object name)
{
return findByProperty(NAME, name);
}


public List findAll()
{
log.debug("finding all Student instances");

try
{
String queryString = "from Student";
Query queryObject = getSession().createQuery(queryString);
return queryObject.list();

} catch (RuntimeException re)
{
log.error("find all failed", re);
throw re;
}
}


public Student merge(Student detachedInstance)
{
log.debug("merging Student instance");

try
{
Student result = (Student) getSession().merge(detachedInstance);
log.debug("merge successful");
return result;

} catch (RuntimeException re)
{
log.error("merge failed", re);
throw re;
}
}


public void attachDirty(Student instance)
{
log.debug("attaching dirty Student instance");

try
{
getSession().saveOrUpdate(instance);
log.debug("attach successful");

} catch (RuntimeException re)
{
log.error("attach failed", re);
throw re;
}
}


public void attachClean(Student instance)
{
log.debug("attaching clean Student instance");

try
{
getSession().lock(instance, LockMode.NONE);
log.debug("attach successful");

} catch (RuntimeException re)
{
log.error("attach failed", re);
throw re;
}
}
}
package com.lusm.stu.vo;


public class Student implements java.io.Serializable
{

private Long id;
private String name;


public Student()
{
}


public Student(Long id, String name)
{
this.id = id;
this.name = name;
}


public Long getId()
{
return this.id;
}


public void setId(Long id)
{
this.id = id;
}


public String getName()
{
return this.name;
}


public void setName(String name)
{
this.name = name;
}

}
Student.hbm.xml
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<!--
Mapping file autogenerated by MyEclipse Persistence Tools
-->
<hibernate-mapping>
<class name="com.lusm.stu.vo.Student" table="STUDENT" schema="LUSM">
<id name="id" type="java.lang.Long">
<column name="ID" precision="22" scale="0" />
<generator class="assigned" />
</id>
<property name="name" type="java.lang.String">
<column name="NAME" length="20" not-null="true" />
</property>
</class>
</hibernate-mapping>
package com.lusm.test;

import java.util.Iterator;
import java.util.List;

import org.hibernate.Query;
import org.hibernate.Session;

import com.lusm.hib.factory.HibernateSessionFactory;
import com.lusm.stu.vo.Student;


public class Test
{

public static void main(String[] args)
{
Session session = HibernateSessionFactory.getSession();
String sql = "from Student";
Query query = session.createQuery(sql);
List list = query.list();
session.close();
Iterator i = list.iterator();
System.out.println("id"+"\t"+"name");
System.out.println("---------------");

while (i.hasNext())
{
Student stu = (Student) i.next();
System.out.println(stu.getId() + "\t" + stu.getName());
}

}
}
hibernate.cfg.xml
<?xml version='1.0' encoding='UTF-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">

<!-- Generated by MyEclipse Hibernate Tools. -->
<hibernate-configuration>

<session-factory>
<property name="connection.username">test</property>
<property name="connection.url">
jdbc:oracle:thin:@localhost:1521:oracle
</property>
<property name="dialect">
org.hibernate.dialect.Oracle9Dialect
</property>
<property name="myeclipse.connection.profile">
Oracle
</property>
<property name="connection.password">123456</property>
<property name="connection.driver_class">
oracle.jdbc.OracleDriver
</property>
<property name="show_sql">true</property>
<mapping resource="com/lusm/stu/vo/Student.hbm.xml" />

</session-factory>

</hibernate-configuration>
运行结果是
log4j:WARN No appenders could be found for logger (org.hibernate.cfg.Environment).
log4j:WARN Please initialize the log4j system properly.
Hibernate: select student0_.ID as ID0_, student0_.NAME as NAME0_ from LUSM.STUDENT student0_
id name
---------------
1 aaaaa
2 bbbbb
3 ccccc
请注意:如果你在运行过程中出现找不到HibernateSessionFactory,或者提示日志文件错误时候,请看下个文章!
地震让大伙知道:居安思危,才是生存之道。
posted on 2007-09-14 21:37
小寻 阅读(873)
评论(0) 编辑 收藏 所属分类:
j2se/j2ee/j2me