大家好啊这一篇,这是我和网友邪逸一起完成的,多亏邪逸,不然我也许还迷糊着画错图了;呵呵
Clock.java 代码
import java.util.*;
import java.awt.*;
import java.applet.*;
import java.text.*;
public class Clock extends Applet implements Runnable {
private volatile Thread timer; // 创建一个可变的线程
private int lastxs, lastys, lastxm,
lastym, lastxh, lastyh; // Dimensions used to draw hands
private SimpleDateFormat formatter; // 创建一个简单数据格式
private String lastdate; // 用于保留最后的数据
private Font clockFaceFont; // 钟面的数字
private Date currentDate; // Used to get date to display
private Color handColor; // Color of main hands and dial
private Color numberColor; // Color of second hand and numbers
private int xcenter = 80, ycenter = 55; // 中间点
public void init() {
int x,y;
lastxs = lastys = lastxm = lastym = lastxh = lastyh = 0;//初始化
formatter = new SimpleDateFormat ("EEE MMM dd hh:mm:ss yyyy",
Locale.getDefault());//初始化前面定义的数据格式
currentDate = new Date();
/*
Date() 在 java.util.Date用于获取当前时间,精确到秒
*/
lastdate = formatter.format(currentDate);//用前面定义的格式,格式化时间变量
clockFaceFont = new Font("Serif", Font.PLAIN, 14);//定义
handColor = Color.blue;//定义分针颜色
numberColor = Color.darkGray; //定义秒针颜色
/*
下面开始定义各个要接收的参数,其实,运行时并没有用到
如果你有兴趣,可以通过定义,html文件上的
<applet code="Clock.class" width=170 height=150>
<param name=bgcolor value="000000">
<param name=fgcolor1 value="ff0000">
<param name=fgcolor2 value="ff00ff">
</applet>
定义要显示的颜色
*/
try {
setBackground(new Color(Integer.parseInt(getParameter("bgcolor"),
16)));
} catch (NullPointerException e) {
} catch (NumberFormatException e) {
}
try {
handColor = new Color(Integer.parseInt(getParameter("fgcolor1"),
16));
} catch (NullPointerException e) {
} catch (NumberFormatException e) {
}
try {
numberColor = new Color(Integer.parseInt(getParameter("fgcolor2"),
16));
} catch (NullPointerException e) {
} catch (NumberFormatException e) {
}
resize(300,300); // 设置时钟窗口大小
}
// 核心代码,实现一个变动的画图,前面讲到的闪光字代码和这个原理有所想近
public void update(Graphics g) {
int xh, yh, xm, ym, xs, ys;
int s = 0, m = 10, h = 10;
String today;
currentDate = new Date();
formatter.applyPattern("s");//这里只解释这个代码,其他照推一样。
try {
s = Integer.parseInt(formatter.format(currentDate));
} catch (NumberFormatException n) {
s = 0;
}
/*
这里先调用formatter.applyPattern("s"),让s的格式化为前面“EEE MMM dd hh:mm:ss yyyy”中的一种格式;
既而使用s = Integer.parseInt(formatter.format(currentDate))获取时间,也许有人会问怎么知道他是小时还是
分钟还是秒;呵呵,这是由前面hh:mm:ss决定的,参数是从后向前取
*/
formatter.applyPattern("m");
try {
m = Integer.parseInt(formatter.format(currentDate));
} catch (NumberFormatException n) {
m = 10;
}
formatter.applyPattern("h");
try {
h = Integer.parseInt(formatter.format(currentDate));
} catch (NumberFormatException n) {
h = 10;
}
// 设置表针最后位置
//这里调用PI圆周率
xs = (int) (Math.cos(s * Math.PI / 30 - Math.PI / 2) * 45 + xcenter);
ys = (int) (Math.sin(s * Math.PI / 30 - Math.PI / 2) * 45 + ycenter);
/*
这里只解释这一个,xs,ys分别是针尾的坐标
看图:
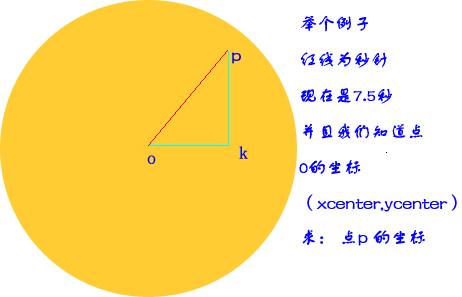
这里只证明线段ok的大小,至于kp就照样画葫芦,容易
这里ok=op×cos<pok=45×cos<pok,所以只要求出cos<pok问题解决
cos<pok的计算使用的钟面的时间7.5,cos<pok=cos(7.5×pi/2 - pi/2)
讲得到的ok 长度加上0点的x 坐标xcenter就得到p点的x坐标了
*/
xm = (int) (Math.cos(m * Math.PI / 30 - Math.PI / 2) * 40 + xcenter);
ym = (int) (Math.sin(m * Math.PI / 30 - Math.PI / 2) * 40 + ycenter);
xh = (int) (Math.cos((h*30 + m / 2) * Math.PI / 180 - Math.PI / 2) * 30
+ xcenter);
yh = (int) (Math.sin((h*30 + m / 2) * Math.PI / 180 - Math.PI / 2) * 30
+ ycenter);
// Get the date to print at the bottom
formatter.applyPattern("EEE MMM dd HH:mm:ss yyyy");
today = formatter.format(currentDate);
g.setFont(clockFaceFont);
// Erase if necessary
g.setColor(getBackground());
if (xs != lastxs || ys != lastys) {//这里判断如果指针发生变动,如果有变动
g.drawLine(xcenter, ycenter, lastxs, lastys);//从新画指针,下面同理
g.drawString(lastdate, 5, 125);
}
if (xm != lastxm || ym != lastym) {
g.drawLine(xcenter, ycenter-1, lastxm, lastym);
g.drawLine(xcenter-1, ycenter, lastxm, lastym);
}
if (xh != lastxh || yh != lastyh) {
g.drawLine(xcenter, ycenter-1, lastxh, lastyh);
g.drawLine(xcenter-1, ycenter, lastxh, lastyh);
}
// 这里写钟面的数字,简单,不解释
g.setColor(numberColor);
g.drawString(today, 5, 125);
g.drawLine(xcenter, ycenter, xs, ys);//设置画指针的参数
g.setColor(handColor);
g.drawLine(xcenter, ycenter-1, xm, ym);
g.drawLine(xcenter-1, ycenter, xm, ym);
g.drawLine(xcenter, ycenter-1, xh, yh);
g.drawLine(xcenter-1, ycenter, xh, yh);
lastxs = xs; lastys = ys;
lastxm = xm; lastym = ym;
lastxh = xh; lastyh = yh;
lastdate = today;
currentDate = null;
}
public void paint(Graphics g) {
g.setFont(clockFaceFont);
// Draw the circle and numbers
g.setColor(handColor);
g.drawArc(xcenter-50, ycenter-50, 100, 100, 0, 360);
g.setColor(numberColor);
g.drawString("9", xcenter-45, ycenter+3);
g.drawString("3", xcenter+40, ycenter+3);
g.drawString("12", xcenter-5, ycenter-37);
g.drawString("6", xcenter-3, ycenter+45);
//到这里画完数字
//开始画我们的时钟指针
g.setColor(numberColor);
g.drawString(lastdate, 5, 125);
g.drawLine(xcenter, ycenter, lastxs, lastys);//op坐标
g.setColor(handColor);
g.drawLine(xcenter, ycenter-1, lastxm, lastym);
g.drawLine(xcenter-1, ycenter, lastxm, lastym);
g.drawLine(xcenter, ycenter-1, lastxh, lastyh);
g.drawLine(xcenter-1, ycenter, lastxh, lastyh);
}
public void start() {
timer = new Thread(this);
timer.start();
}
public void stop() {
timer = null;
}
public void run() {
Thread me = Thread.currentThread();
while (timer == me) {
try {
Thread.currentThread().sleep(100);//这里用的是毫秒100=1秒
} catch (InterruptedException e) {
}
repaint();
}
}
}
index.htm代码:
<HTML>
<HEAD>
<TITLE>A Clock (1.6)</TITLE>
</HEAD>
<BODY>
<h1>A Clock (1.6)</h1>
<hr>
<applet code="Clock.class" width=170 height=150>
alt="Your browser understands the <APPLET> tag but isn't running the applet, for some reason."
Your browser is completely ignoring the <APPLET> tag!
</applet>
<p>
The clock applet now has three parameters; the background
color (bgcolor), the main foreground color (the hands and
dial) (fgcolor1) and the secondary foreground color (the
seconds hand and numbers) (fgcolor2). These three parameters
are hexadecimal RGB numbers (like the ones used for the body
bgcolor tag in HTML). For example:
<p>
<applet code="Clock.class" width=170 height=150><br>
<param name=bgcolor value="000000"><br>
<param name=fgcolor1 value="ff0000"><br>
<param name=fgcolor2 value="ff00ff"><br>
</applet><p>
would give you a black background, a red dial and hands, and purple numbers.
<p>
For those who don't convert to hexadecimal easily, here are some common
values:
<ul>
<li>black = 000000
<li>blue = 0000ff
<li>cyan = 00ffff
<li>darkGray = 404040
<li>gray = 808080
<li>green = 00ff00
<li>lightGray = c0c0c0
<li>magenta = ff00ff
<li>orange = ffc800
<li>pink = ffafaf
<li>red = ff0000
<li>white = ffffff
<li>yellow = ffff00
</ul>
<hr>
<a href="Clock.java">The source</a>.
</BODY>
</HTML>
地震让大伙知道:居安思危,才是生存之道。
posted on 2007-03-16 23:41
小寻 阅读(892)
评论(1) 编辑 收藏 所属分类:
j2se/j2ee/j2me