Wicket Lab2 要求使用 TextField, DropDownChoice 及 Date picker 實作頁面跳轉的效果.
開始備忘記:
[1] Lab2 要求
[2] 實作 Lab2
[1] Lab2 要求:
本次要求如下圖所示,
當介面選擇 Male 後, 跳至 male 的訊息,
當介面選擇 Female 後, 跳至 female 的訊息,
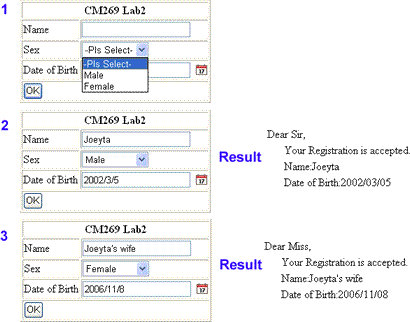
[2] 實作 Lab2
<!----------- web.xml ----------------->
<?xml version="1.0" encoding="UTF-8"?>
<web-app id="WebApp_ID" version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>CM269</display-name>
<filter>
<filter-name>WicketLab1First</filter-name>
<filter-class>org.apache.wicket.protocol.http.WicketFilter</filter-class>
<init-param>
<param-name>applicationClassName</param-name>
<param-value>cm269.lab1.MyApp</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>WicketLab1First</filter-name>
<url-pattern>/lab1/*</url-pattern>
</filter-mapping>
<filter>
<filter-name>WicketLab2First</filter-name>
<filter-class>org.apache.wicket.protocol.http.WicketFilter</filter-class>
<init-param>
<param-name>applicationClassName</param-name>
<param-value>cm269.lab2.MyApp</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>WicketLab2First</filter-name>
<url-pattern>/lab2/*</url-pattern>
</filter-mapping>
</web-app>
<!----------- web.xml ----------------->
############### Lab2.properties ###############
f.sex.null=-Pls Select-
f.birth.IConverter.Date=You must input a date.
############### Lab2.properties ###############
這裡的 f.sex.null 表示 html 頁裡 "f" form 的 sex element,
表示 DropDownChoice 預設為 -Pls Select-
如果 key/value 設成 null=-Pls Select- 則對所有 DropDownChoice 起作用.
/************* Lab2.java ****************/
package cm269.lab2;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.apache.wicket.extensions.yui.calendar.DatePicker;
import org.apache.wicket.markup.html.WebPage;
import org.apache.wicket.markup.html.form.DropDownChoice;
import org.apache.wicket.markup.html.form.Form;
import org.apache.wicket.markup.html.form.TextField;
import org.apache.wicket.markup.html.panel.FeedbackPanel;
import org.apache.wicket.model.Model;
public class Lab2 extends WebPage {
private static final long serialVersionUID = 381689366528324660L;
private Model nameModel;
private Model sexModel;
private Model birthModel;
public Lab2() {
FeedbackPanel feedback = new FeedbackPanel("msgs");
add(feedback);
Form form = new Form("f"){
private static final long serialVersionUID = -8897899191585355738L;
protected void onSubmit() {
String sName = (String)nameModel.getObject();
String sSex = (String)sexModel.getObject();
Date dBirth = (Date)birthModel.getObject();
if(sSex.equals("Male")){
AbstractResult result = new Lab2ResultA(sName,dBirth);
setResponsePage(result); // 這裡表示按 submit 後, 跳至 Lab2ResultA 頁面
} else if(sSex.equals("Female")){
AbstractResult result = new Lab2ResultB(sName,dBirth);
setResponsePage(result); // 這裡表示按 submit 後, 跳至 Lab2ResultB 頁面
}
}
};
nameModel = new Model();
TextField name = new TextField("name", nameModel, String.class);
name.setRequired(true);
form.add(name);
sexModel = new Model();
List sexes = new ArrayList();
sexes.add("Male");
sexes.add("Female");
DropDownChoice sex = new DropDownChoice("sex", sexModel, sexes);
sex.setRequired(true);
form.add(sex);
birthModel = new Model();
TextField birth = new TextField("birth", birthModel, Date.class);
// TextField birth = new TextField("birth", birthModel, Date.class){
// private static final long serialVersionUID = 1441781496720134137L;
// public IConverter getConverter(Class type){
// return type == Date.class ? new DateConverter():null;
// }
// };
birth.setRequired(true);
birth.add(new DatePicker());
form.add(birth);
add(form);
}
}
/************* Lab2.java ****************/
<!----------- Lab2.html ----------------->
<html>
<head>
<title>Lab2</title>
</head>
<body>
<span wicket:id="msgs" />
<form wicket:id="f">
<table border>
<tr><th colspan="2">CM269 Lab2</th></tr>
<tr>
<td>Name</td>
<td>
<input type="text" wicket:id="name" />
</td>
</tr>
<tr>
<td>Sex</td>
<td>
<select wicket:id="sex">
<option>Male</option>
<option>Female</option>
</select>
</td>
</tr>
<tr>
<td>Date of Birth</td>
<td>
<input type="text" wicket:id="birth" />
</td>
</tr>
<tr>
<td colspan="2">
<input type="submit" value="OK" />
</td>
</tr>
</table>
</form>
</body>
</html>
<!----------- Lab2.html ----------------->
/************* AbstractResult.java ****************/
package cm269.lab2;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.wicket.markup.html.WebPage;
import org.apache.wicket.markup.html.basic.Label;
public class AbstractResult extends WebPage{
private static final long serialVersionUID = 3148730572565560427L;
protected String name;
protected Date birth;
public void doResult() {
DateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd");
add(new Label("name",getName()));
add(new Label("birth",dateFormat.format(getBirth())));
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
}
/************* AbstractResult.java ****************/
/************* Lab2ResultA.java ****************/
package cm269.lab2;
import java.util.Date;
public class Lab2ResultA extends AbstractResult {
private static final long serialVersionUID = -1230297067586624251L;
public Lab2ResultA(String sName, Date dBirth) {
setName(sName);
setBirth(dBirth);
doResult();
}
}
/************* Lab2ResultA.java ****************/
<!----------- Lab2ResultA.html ----------------->
<html>
<head>
<title>Lab2ResultA</title>
</head>
<body>
<table border="0">
<tr>
<td colspan="2">Dear Sir,</td>
</tr>
<tr>
<td width="20"> </td>
<td>Your Registration is accepted.</td>
</tr>
<tr>
<td> </td>
<td>Name:<span wicket:id="name">joeyta</span></td>
</tr>
<tr>
<td> </td>
<td>Date of Birth:<span wicket:id="birth">1980/01/01</span></td>
</tr>
</table>
</body>
</html>
<!----------- Lab2ResultA.html ----------------->
/************* Lab2ResultB.java ****************/
package cm269.lab2;
import java.util.Date;
public class Lab2ResultB extends AbstractResult {
private static final long serialVersionUID = -5461297645709489576L;
public Lab2ResultB(String sName, Date dBirth) {
setName(sName);
setBirth(dBirth);
doResult();
}
}
/************* Lab2ResultB.java ****************/
<!----------- Lab2ResultB.html ----------------->
<html>
<head>
<title>Lab2ResultB</title>
</head>
<body>
<table border="0">
<tr>
<td colspan="2">Dear Miss,</td>
</tr>
<tr>
<td width="20"> </td>
<td>Your Registration is accepted.</td>
</tr>
<tr>
<td> </td>
<td>Name:<span wicket:id="name">joeyta</span></td>
</tr>
<tr>
<td> </td>
<td>Date of Birth:<span wicket:id="birth">1980/01/01</span></td>
</tr>
</table>
</body>
</html>
<!----------- Lab2ResultB.html ----------------->
項目結構如下圖所示:
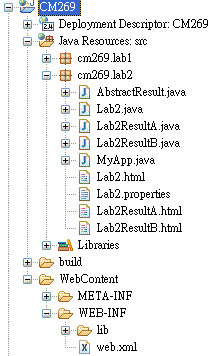
參考資料:
http://wicket.apache.org/