Problem: Started with a simple SimUDuck App..
Joe's company makes a duck pond simulation game, SimUDuck, The game can show a large variety of duck species swimming and making quacking sounds.
Initial Design:

But now some new functionality should be added, for example: we need some of the ducks to FLY.
First Design:
We add a method fly() into the Duck class. It seems worked, but something went horribly wrong because
not all ducks can fly. so....
Second Design: Using inheritance and polymorphism
Always override the fly() mehtod in the subclass where needed.
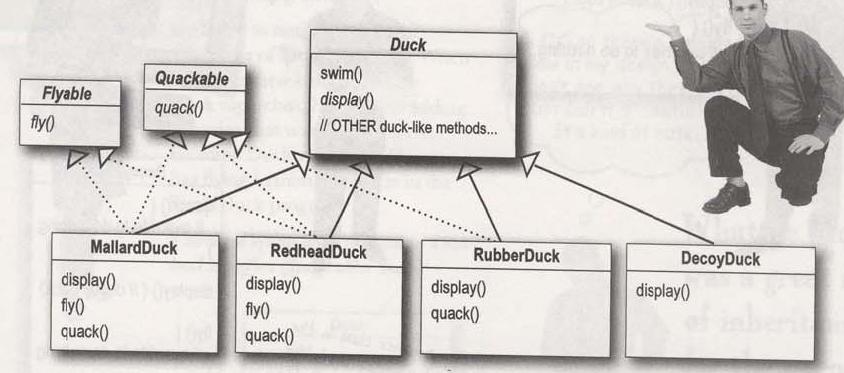
Drawbacks: Everytime a new duck is added, you will be forced to look at and possibly override fly() and quack(). so is there a cleaner way of having only some of the duck types fly or quack?
Third Design: Using interface!

Drawbacks: It completely destroy code reuse for those behaviors.
1. Design Principles: Identify the aspects of your application that vary and separate them form what stays the same! which means Encapsulate the parts that vary!
2. Design Principles: Program to an interface, not an implementation! (interface here means supertype! including interface
and abstract class!.. making use of the polymorphism functionality).
3. Design Principles: Favor composition over interface!
Strategy Pattern: Using Composition!
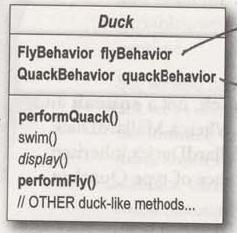
Code implement:
FlyBehavior.java

public interface FlyBehavior
{
public void fly();
}FlyWithWings.java

public class FlyWithWings implements FlyBehavior
{

public void fly()
{
System.out.println("I'm flying!!");
}
}FlyNoWay.java

public class FlyNoWay implements FlyBehavior
{

public void fly()
{
System.out.println("I can't fly");
}
}Duck.java

public abstract class Duck
{
FlyBehavior flyBehavior;

public Duck()
{
}
public abstract void display();

public void performFly()
{
flyBehavior.fly();
}

public void swim()
{
System.out.println("All ducks float, even decoys!");
}
}MallardDuck.java

public class MallardDuck
{

public MallardDuck()
{
flyBehavior=new FlyWithWings();
}

public void display()
{
System.out.println("I'm a real mallard duck");
}
}The Definition of Strategy Pattern: The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them intercahgeable. Strategy lets the algorithm vary indepanedtl from client that use it
Problems:
1. It's weird to have a class that's jast a behavior: classes represent things both have state and methods. a flying behavior might have instance variables representing the attributes for the flying behavior.
2. Be care of Over-Design: implement your code first, then refractoring!