题目是要求描述算术表达式,操作符仅限于+,-,*,/四种二元操作符,操作数仅限于整数。
回来想了一下这个题其实是可以用composite模式来做的,UML静态类图如下:
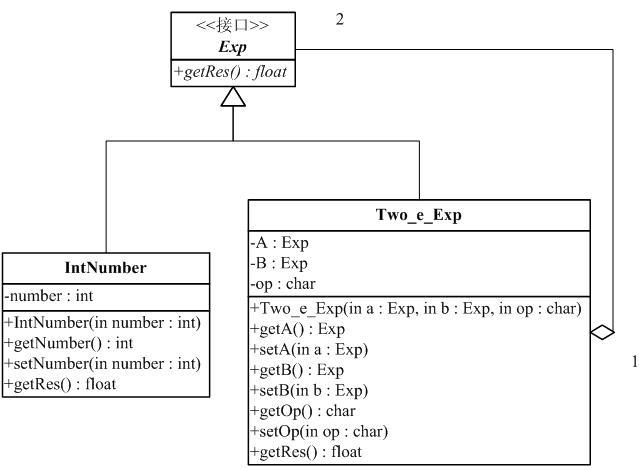
相应的Java代码如下(没有考虑除数为零的异常):
1
interface Exp
{
2
float getRes();
3
}
1
public class Two_e_Exp implements Exp
{
2
private Exp A;
3
4
private Exp B;
5
6
private char op;
7
8
/** *//**
9
* @param a
10
* @param b
11
* @param op
12
*/
13
public Two_e_Exp(Exp a, Exp b, char op)
{
14
A = a;
15
B = b;
16
this.op = op;
17
}
18
19
/** *//**
20
* @return a
21
*/
22
public Exp getA()
{
23
return A;
24
}
25
26
/** *//**
27
* @param a
28
* 要设置的 a
29
*/
30
public void setA(Exp a)
{
31
A = a;
32
}
33
34
/** *//**
35
* @return b
36
*/
37
public Exp getB()
{
38
return B;
39
}
40
41
/** *//**
42
* @param b
43
* 要设置的 b
44
*/
45
public void setB(Exp b)
{
46
B = b;
47
}
48
49
/** *//**
50
* @return op
51
*/
52
public char getOp()
{
53
return op;
54
}
55
56
/** *//**
57
* @param op
58
* 要设置的 op
59
*/
60
public void setOp(char op)
{
61
this.op = op;
62
}
63
64
public float getRes()
{
65
// case '+'
66
float res = A.getRes() + B.getRes();
67
switch (op)
{
68
case '-':
{
69
res = A.getRes() - B.getRes();
70
break;
71
}
72
case '*':
{
73
res = A.getRes() * B.getRes();
74
break;
75
}
76
case '/':
{
77
res = A.getRes() / B.getRes();
78
break;
79
}
80
}
81
return res;
82
}
83
84
}
1
public class IntNumber implements Exp
{
2
private int number;
3
4
/** *//**
5
* @param number
6
*/
7
public IntNumber(int number)
{
8
this.number = number;
9
}
10
11
/** *//**
12
* @return number
13
*/
14
public int getNumber()
{
15
return number;
16
}
17
18
/** *//**
19
* @param number 要设置的 number
20
*/
21
public void setNumber(int number)
{
22
this.number = number;
23
}
24
25
public float getRes()
{
26
return number;
27
}
28
29
}
一个简单的测试程序:
1
public class Main
{
2
3
/** *//**
4
* @param args
5
*/
6
public static void main(String[] args)
{
7
Exp e1 =new Two_e_Exp(new IntNumber(1),new IntNumber(2),'+');
8
Exp e2 =new Two_e_Exp(new IntNumber(3),new IntNumber(4),'*');
9
Exp e =new Two_e_Exp(e2,e1,'/');
10
// (3*4)/(1+2)=4
11
System.out.println(e.getRes());
12
((Two_e_Exp)e1).setA(new IntNumber(2));
13
// (3*4)/(2+2)=3
14
System.out.println(e.getRes());
15
((Two_e_Exp)e).setA(new Two_e_Exp(new IntNumber(12),new IntNumber(12),'+'));
16
//(12+12)/(2+2)=6
17
System.out.println(e.getRes());
18
//(12+12)/(2+(5-3))=6
19
((Two_e_Exp)e1).setB(new Two_e_Exp(new IntNumber(5),new IntNumber(3),'-'));
20
System.out.println(e.getRes());
21
}
22
23
}