//html代码
<div id="container"></div>
//js代码
 var p = new Ext.Panel( {
title: 'My Panel',//标题
collapsible:true,//右上角上的那个收缩按钮,设为false则不显示
renderTo: 'container',//这个panel显示在html中id为container的层中
width:400,
height:200,
html: "<p>我是内容,我包含的html可以被执行!</p>"//panel主体中的内容,可以执行html代码
});
因为panel组件的子类组件包括TabPanel,GridPanel,FormPanel,TreePanel组件,所以非常有必要介绍Panel组件的配置参数和相关的属性、方法。
//配置参数(只列举部分常用参数)
1.autoLoad:有效的url字符串,把那个url中的body中的数据加载显示,但是可能没有样式和js控制,只是html数据
2.autoScroll:设为true则内容溢出的时候产生滚动条,默认为false
3.autoShow:设为true显示设为"x-hidden"的元素,很有必要,默认为false

4.bbar:底部条,显示在主体内,//代码:bbar:[{text:'底部工具栏bottomToolbar'}],
5.tbar:顶部条,显示在主体内,//代码:tbar:[{text:'顶部工具栏topToolbar'}],
6.buttons:按钮集合,自动添加到footer中(footer参数,显示在主体外)//代码:buttons:[{text:"按钮位于footer"}]
7.buttonAlign:footer中按钮的位置,枚举值为:"left","right","center",默认为right

8.collapsible:设为true,显示右上角的收缩按钮,默认为false
9.draggable:true则可拖动,但需要你提供操作过程,默认为false

10.html:主体的内容
11.id:id值,通过id可以找到这个组件,建议一般加上这个id值
12.width:宽度
13.height:高度
13.title:标题

14.titleCollapse:设为true,则点击标题栏的任何地方都能收缩,默认为false.

15.applyTo:(id)呈现在哪个html元素里面
16.contentEl:(id)呈现哪个html元素里面,把el内的内容呈现
17.renderTo:(id)呈现在哪个html元素里面
//关于这三个参数的区别(个人认为:applyTo和RenderTo强调to到html元素中,contentEl则是html元素到ext组件中去):
英文如下(本人英语poor,不敢乱翻译):
contentEl - This config option is used to take existing content and place it in the body of a new panel. It is not going to be the actual panel itself. (It will actually copy the innerHTML of the el and use it for the body). You should add either the x-hidden or the x-hide-display CSS class to prevent a brief flicker of the content before it is rendered to the panel.
applyTo - This config option allows you to use pre-defined markup to create an entire Panel. By entire, I mean you can include the header, tbar, body, footer, etc. These elements must be in the correct order/hierarchy. Any components which are not found and need to be created will be autogenerated.
renderTo - This config option allows you to render a Panel as its created. This would be the same as saying myPanel.render(ELEMENT_TO_RENDER_TO);
哪位大人帮忙翻译下
18 frame: true,//圆角边框
拖动的例子:
//下面创建一个允许拖动的panel,但是拖动的结果不能保存
 var p=new Ext.Panel( {
title: 'Drag me',
x: 100,
y: 100,
renderTo: Ext.getBody(),//x,y,renderTo:Ext.getBody()初始化panel的位置
floating: true,//true
frame: true,//圆角边框
width: 400,
height: 200,
draggable:true
}).show();//在这里也可以不show()
但是还不能拖到其他的地方,我们需要改写draggable:
 draggable: {
insertProxy: false,//拖动时不虚线显示原始位置

 onDrag : function(e) {
var pel = this.proxy.getEl();
this.x = pel.getLeft(true);
this.y = pel.getTop(true);//获取拖动时panel的坐标
},

 endDrag : function(e) {
this.panel.setPosition(this.x, this.y);//移动到最终位置
}
}
实现了可保存的拖动,如图:
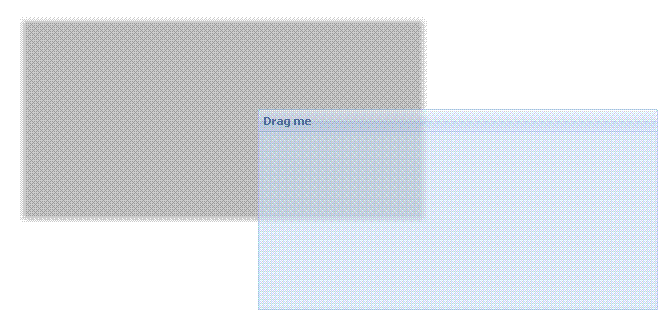
拖动的时候阴影还在原位置,我们再在draggable中的onDrag事件中添加代码:
var s = this.panel.getEl().shadow;
 if (s) {
s.realign(this.x, this.y, pel.getWidth(), pel.getHeight());
}
//shadow的realign方法的四个参数,改变shadow的位置大小属性
最后这个可拖动的panel的代码为:
 var p=new Ext.Panel( {
title: 'Drag me',
x: 100,
y: 100,
renderTo: Ext.getBody(),
floating: true,
frame: true,
width: 400,
height: 200,
 draggable: {
insertProxy: false,

 onDrag : function(e) {
var pel = this.proxy.getEl();
this.x = pel.getLeft(true);
this.y = pel.getTop(true);

var s = this.panel.getEl().shadow;
 if (s) {
s.realign(this.x, this.y, pel.getWidth(), pel.getHeight());
}
},
 endDrag : function(e) {
this.panel.setPosition(this.x, this.y);
}
}
})

2.带顶部,底部,脚部工具栏的panel
 var p=new Ext.Panel( {
id:"panel1",
title:"标题",
collapsible:true,
renderTo:"container",
closable:true,
width:400,
height:300,
 tbar:[ {text:"按钮1"}, {text:"按钮2"}], //顶部工具栏
 bbar:[ {text:"按钮1"}, {text:"按钮2"}], //底部工具栏
html:"内容",
 buttons:[ {text:"按钮1"}, {text:"按钮2"}] //footer部工具栏
});
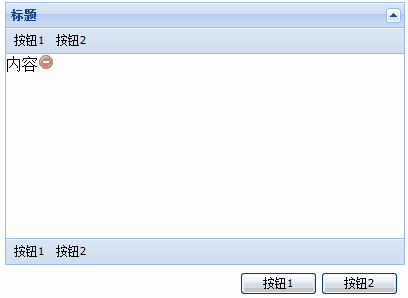
我们已经在各种工具栏上添加了按钮,但是却没有激发事件,下面我们来添加按钮事件代码:
 tbar:[ {text:"按钮1",handler:function() {Ext.MessageBox.alert("我是按钮1","我是通过按钮1激发出来的弹出框!")}}, {text:"按钮2"}],
//改写tbar,添加handler句柄,点击顶部工具栏上按钮1,弹出提示框,效果图大家想象下,就不贴出来了
3.panel工具栏
//添加下面的代码到panel配置参数中
 tools:[ {id:"save"}, {id:"help"}, {id:"up"}, {id:"close",handler:function() {Ext.MessageBox.alert("工具栏按钮","工具栏上的关闭按钮时间被激发了")}}],
//id控制按钮,handler控制相应的事件
// id的枚举值为:
toggle (collapsable为true时的默认值)
close
minimize
maximize
restore
gear
pin
unpin
right
left
up
down
refresh
minus
plus
help
search
save
print
|